An automobile 4S shop sells SUV and family car models. SUV is sold at a discount of 95% of the manufacturer's guide price, and family car is sold at a direct price reduction of 18000 yuan. Please construct a VehicleSale abstract base class, in which a pure virtual function SalePrice is defined to calculate the actual sales price. Two classes, SUVSale and CarSale, are derived from the VehicleSale class.
时间: 2023-07-02 17:19:47 浏览: 74
Sure, I can help you with that. Here's an example code that implements the abstract base class and its derived classes:
```cpp
#include <iostream>
using namespace std;
// VehicleSale abstract base class
class VehicleSale {
public:
virtual double SalePrice(double guide_price) = 0; // pure virtual function
};
// SUVSale derived class
class SUVSale : public VehicleSale {
public:
double SalePrice(double guide_price) {
return guide_price * 0.95; // 5% discount
}
};
// CarSale derived class
class CarSale : public VehicleSale {
public:
double SalePrice(double guide_price) {
return guide_price - 18000; // direct price reduction
}
};
// main function for testing
int main() {
double suv_guide_price = 50000;
double car_guide_price = 80000;
SUVSale suv_sale;
CarSale car_sale;
cout << "SUV sale price: " << suv_sale.SalePrice(suv_guide_price) << endl;
cout << "Car sale price: " << car_sale.SalePrice(car_guide_price) << endl;
return 0;
}
```
In this example, the `VehicleSale` class is defined as an abstract base class with a pure virtual function `SalePrice`. This function takes the manufacturer's guide price as input and returns the actual sales price.
The `SUVSale` and `CarSale` classes are derived from `VehicleSale` and implement their own `SalePrice` functions based on the specific sale strategies. `SUVSale` applies a 5% discount to the guide price, while `CarSale` simply reduces the price by 18000 yuan.
In the `main` function, we create objects of `SUVSale` and `CarSale` and call their `SalePrice` functions to calculate the actual sale prices for a given guide price. The results are printed to the console for testing purposes.
相关推荐
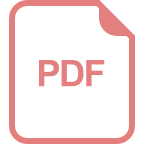
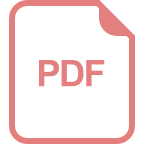
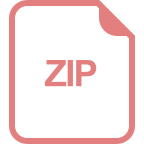














