请使用标准C++库实现的WAV音频文件读写
时间: 2024-03-07 14:47:46 浏览: 24
好的,下面是使用标准C++库实现的WAV音频文件读写的示例:
```c++
#include <iostream>
#include <fstream>
using namespace std;
// WAV文件头信息结构体
struct WAVHeader {
char riff[4]; // "RIFF"
int fileSize; // 文件总大小
char wave[4]; // "WAVE"
char fmt[4]; // "fmt "
int fmtSize; // fmt块大小
short audioFormat; // 音频格式
short numChannels; // 声道数
int sampleRate; // 采样率
int byteRate; // 码率
short blockAlign; // 区块对齐
short bitsPerSample; // 每个样本的位数
char data[4]; // "data"
int dataSize; // 数据大小
};
int main() {
// 读取WAV文件
ifstream fin("test.wav", ios::binary);
if (!fin) {
cout << "打开文件失败!" << endl;
return 1;
}
WAVHeader header;
fin.read((char*)&header, sizeof(header));
if (strncmp(header.riff, "RIFF", 4) != 0 || strncmp(header.wave, "WAVE", 4) != 0 || strncmp(header.fmt, "fmt ", 4) != 0 || strncmp(header.data, "data", 4) != 0) {
cout << "不是有效的WAV文件!" << endl;
return 1;
}
cout << "音频格式:" << header.audioFormat << endl;
cout << "声道数:" << header.numChannels << endl;
cout << "采样率:" << header.sampleRate << endl;
cout << "码率:" << header.byteRate << endl;
cout << "每个样本的位数:" << header.bitsPerSample << endl;
cout << "数据大小:" << header.dataSize << endl;
// 读取音频数据
char* data = new char[header.dataSize];
fin.read(data, header.dataSize);
fin.close();
// 写入WAV文件
ofstream fout("output.wav", ios::binary);
if (!fout) {
cout << "创建文件失败!" << endl;
return 1;
}
fout.write((char*)&header, sizeof(header));
fout.write(data, header.dataSize);
fout.close();
delete[] data;
return 0;
}
```
这个示例使用了标准C++库中的ifstream和ofstream类读写文件,并使用WAVHeader结构体来存储WAV文件头信息。通过读取WAV文件头信息,可以获取音频格式、声道数、采样率、码率、每个样本的位数和数据大小等信息,然后读取音频数据并写入新的WAV文件中。
相关推荐
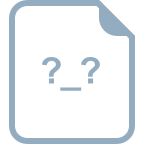
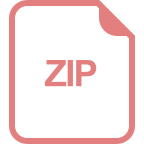
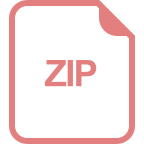
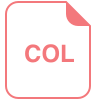













