写一篇C++互斥锁要点的应用文章
时间: 2023-03-21 08:02:23 浏览: 114
C语言中的互斥锁是一种常用的同步工具,用于保护多线程应用程序中共享资源的访问。下面是使用互斥锁的要点和示例应用。
一、互斥锁的要点
1. 创建互斥锁:使用pthread_mutex_init()函数初始化一个互斥锁变量。
2. 锁定互斥锁:使用pthread_mutex_lock()函数锁定一个互斥锁。如果互斥锁已经被锁定,则调用线程将阻塞,直到互斥锁被释放。
3. 解锁互斥锁:使用pthread_mutex_unlock()函数解锁一个互斥锁。
4. 销毁互斥锁:使用pthread_mutex_destroy()函数销毁一个互斥锁。
二、示例应用
下面是一个示例应用程序,演示了如何使用互斥锁来保护多线程应用程序中的共享资源。
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define NUM_THREADS 10
pthread_mutex_t mutex;
int count = 0;
void *thread_func(void *arg)
{
int i;
for (i = 0; i < 1000000; i++)
{
pthread_mutex_lock(&mutex);
count++;
pthread_mutex_unlock(&mutex);
}
pthread_exit(NULL);
}
int main()
{
int i;
pthread_t threads[NUM_THREADS];
pthread_mutex_init(&mutex, NULL);
for (i = 0; i < NUM_THREADS; i++)
{
pthread_create(&threads[i], NULL, thread_func, NULL);
}
for (i = 0; i < NUM_THREADS; i++)
{
pthread_join(threads[i], NULL);
}
pthread_mutex_destroy(&mutex);
printf("count = %d\n", count);
return 0;
}
在这个示例程序中,我们创建了一个互斥锁变量mutex,并定义了一个共享变量count。然后,我们创建了10个线程,每个线程都会对count变量进行1000000次自增操作。在这个操作中,我们使用互斥锁来保护count变量的访问,确保每次自增操作都是原子的。最后,我们输出count变量的值,验证程序的正确性。
通过这个示例应用程序,我们可以看到互斥锁的使用方法和应用场景。在多线程应用程序中,使用互斥锁是一种有效的方式来保护共享资源的访问,避免竞态条件和数据损坏的问题。
阅读全文
相关推荐
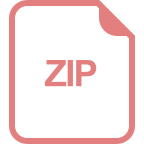
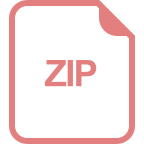
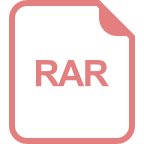
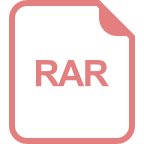
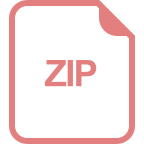
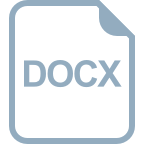
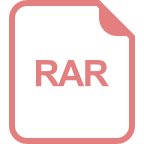
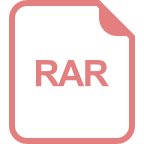
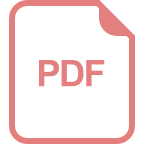
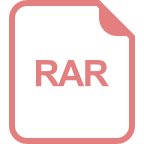
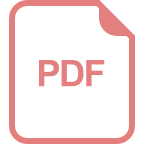
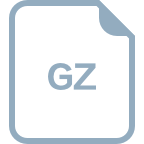
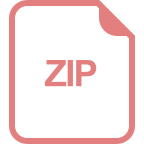
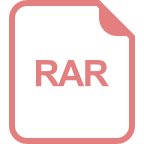