redistemplate设置increment的同时设置时间
时间: 2023-02-08 14:15:56 浏览: 184
在 Redis 中,使用 `INCR` 命令来对 key 执行递增操作。如果想要在递增的同时设置时间,可以使用 `INCRBY` 命令,格式如下:
```
INCRBY key increment
```
其中,key 是需要递增的键,increment 是要增加的值。
此外,如果你需要限制键的过期时间,可以在这个命令之后使用 `EXPIRE` 命令来设置。例如,设置过期时间为120s
```
EXPIRE key 120
```
注意,如果 key 不存在,那么执行 INCRBY 命令时,会自动创建一个新的 key,并将其值设置为 increment。
通过这种方式,可以在一个命令中同时设置 key 的值和过期时间。
相关问题
springboot操作redis的increment方法同时设置时间
在Spring Boot中,可以使用RedisTemplate来操作Redis,并且可以通过RedisTemplate的opsForValue()方法来获取ValueOperations对象,进而操作Redis中的值。要实现increment方法同时设置过期时间,可以使用ValueOperations的increment方法和expire方法结合起来使用,示例如下:
```java
@Autowired
private RedisTemplate<String, Integer> redisTemplate;
public void incrementWithExpire(String key, int delta, long expireSeconds) {
ValueOperations<String, Integer> ops = redisTemplate.opsForValue();
ops.increment(key, delta);
redisTemplate.expire(key, expireSeconds, TimeUnit.SECONDS);
}
```
在上述代码中,通过redisTemplate注入RedisTemplate对象,并且指定泛型为<String, Integer>,表示操作的是Redis中的字符串类型,并且存储的值为Integer类型。incrementWithExpire方法中,首先通过opsForValue()方法获取ValueOperations对象,然后调用increment方法实现对key的值进行增加操作,最后调用expire方法设置key的过期时间。其中expireSeconds表示过期时间,单位为秒。
org.springframework.data.redis.core.ValueOperations#increment 设置过期时间
是的,可以通过调用`ValueOperations#increment`方法的重载版本来设置过期时间。例如,使用以下代码可以将key的值递增1,并在60秒后过期:
```
ValueOperations<String, String> ops = redisTemplate.opsForValue();
ops.increment("key", 1L);
redisTemplate.expire("key", 60, TimeUnit.SECONDS);
```
其中,`redisTemplate`是RedisTemplate实例,类型参数分别为String和String。`ops.increment`方法的第二个参数指定递增的数量,这里为1L。`redisTemplate.expire`方法用于设置过期时间,第一个参数为key,第二个参数为过期时间,第三个参数为时间单位。
阅读全文
相关推荐
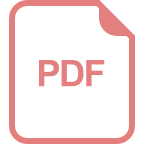
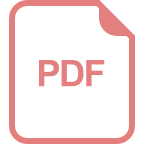
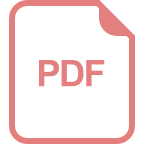













