Zombie Apocalypse 用typescript
时间: 2024-05-16 22:17:05 浏览: 141
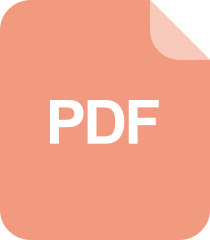
typescript
好的,让我们来创建一个用 TypeScript 编写的 Zombie Apocalypse 游戏。
首先,我们需要定义一些游戏中会用到的类型。例如,我们需要定义一个 `Player` 类型来代表游戏中的玩家:
```typescript
interface Player {
name: string;
health: number;
attack: number;
defense: number;
}
```
接下来,我们需要定义一个 `Zombie` 类型来代表游戏中的僵尸:
```typescript
interface Zombie {
health: number;
attack: number;
defense: number;
}
```
然后,我们需要定义一个 `Game` 类来管理游戏状态和逻辑:
```typescript
class Game {
private player: Player;
private zombies: Zombie[];
constructor(player: Player, zombies: Zombie[]) {
this.player = player;
this.zombies = zombies;
}
play() {
while (this.player.health > 0 && this.zombies.length > 0) {
const zombie = this.zombies[0];
const playerDamage = this.player.attack - zombie.defense;
const zombieDamage = zombie.attack - this.player.defense;
if (playerDamage > 0) {
zombie.health -= playerDamage;
}
if (zombie.health <= 0) {
this.zombies.shift();
}
if (zombieDamage > 0) {
this.player.health -= zombieDamage;
}
}
if (this.player.health > 0) {
console.log('You win!');
} else {
console.log('You lose!');
}
}
}
```
最后,我们可以创建一个游戏实例并开始游戏:
```typescript
const player: Player = {
name: 'John',
health: 100,
attack: 10,
defense: 5,
};
const zombies: Zombie[] = [
{ health: 20, attack: 5, defense: 2 },
{ health: 30, attack: 7, defense: 3 },
{ health: 40, attack: 10, defense: 4 },
];
const game = new Game(player, zombies);
game.play();
```
这就是用 TypeScript 编写的 Zombie Apocalypse 游戏。当然,你可以根据自己的喜好和需求对代码进行修改和扩展。
阅读全文
相关推荐
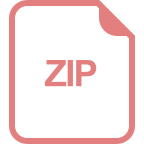
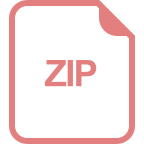
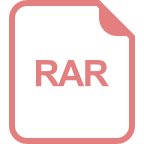
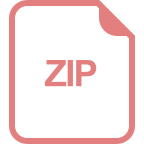
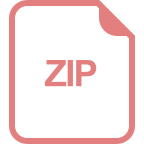
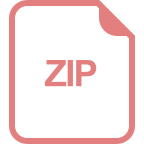
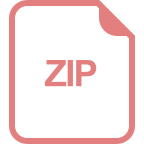
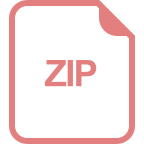
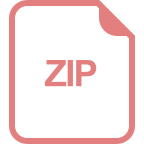
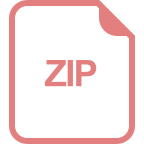
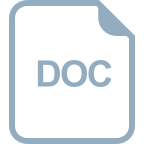
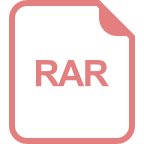
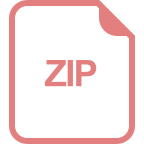
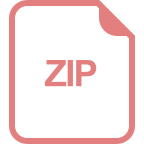
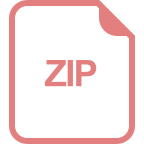
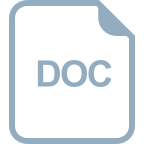
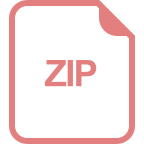
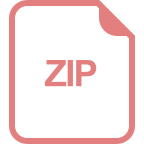