What is the recursion for computing the sum of the squares of the first n natural numbers? Denote it by Q(n).
时间: 2024-05-30 19:11:34 浏览: 13
The recursion for computing the sum of the squares of the first n natural numbers is:
Q(n) = Q(n-1) + n^2
with initial condition Q(0) = 0.
In other words, to compute Q(n), we can first compute Q(n-1) and then add n^2 to it. This process continues until we reach the base case Q(0), which is defined to be 0.
相关问题
Write a program in C to calculate the sum of numbers from 1 to n using recursion. Input: Input a positve integer n. Output : Compute the summation of 1+2+...+n. Sample of Input: 5 Sample of Output: 15
Sure, I can help you with that. Here's the C program to calculate the sum of numbers from 1 to n using recursion:
```c
#include <stdio.h>
int sum(int n);
int main() {
int n, result;
printf("Enter a positive integer: ");
scanf("%d", &n);
result = sum(n);
printf("The sum of numbers from 1 to %d is %d.\n", n, result);
return 0;
}
int sum(int n) {
if (n == 0) {
return 0;
} else {
return n + sum(n-1);
}
}
```
Explanation:
The program takes a positive integer `n` as input from the user and calls the `sum()` function with `n` as argument.
The `sum()` function is a recursive function that takes an integer `n` as input and returns the sum of numbers from 1 to `n`.
If `n` is 0, the function returns 0. Otherwise, it adds `n` to the sum of numbers from 1 to `n-1` by calling itself recursively with `n-1` as argument.
Finally, the program outputs the result, which is the sum of numbers from 1 to `n`.
maximum recursion depth exceeded while getting the repr of an object
这个错误通常在递归函数中出现,表示递归层数过多,导致 Python 解释器崩溃。最常见的原因是在递归函数中没有正确设置递归终止条件,导致函数不断地递归调用自己,直到超出 Python 解释器的最大递归深度。解决方法是检查递归函数的终止条件是否正确设置,并考虑优化算法以减少递归深度。如果确实需要更深的递归深度,可以通过设置 sys.setrecursionlimit() 来增加 Python 解释器的最大递归深度。但是,这种做法并不推荐,因为可能会导致程序出现其他问题。
相关推荐
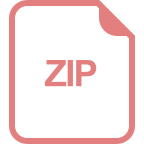
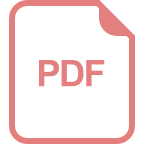
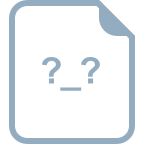













