C++通过欧姆龙 EntherNet/IP CIP报文批量写入示例代码
时间: 2023-10-06 09:05:12 浏览: 374
欧姆龙的 EntherNet/IP CIP 协议是一种用于工业自动化领域的通信协议,它可以实现不同设备之间的数据通信。在 C++ 中,可以通过使用开源的库来实现对 EntherNet/IP CIP 协议的支持。以下是一个示例代码,演示如何使用 EntherNet/IP CIP 报文批量写入数据:
```c++
#include <iostream>
#include <cstring>
#include "cipster_api.h"
#include "cipster_user_conf.h"
using namespace std;
int main()
{
CipsterApi::Init();
// 设备的 IP 地址和端口号
const char* ip_address = "192.168.0.1";
int port = 0xAF12;
// 创建一个连接对象
Connection connection;
connection.originator_address = kCipAnyIpAddress; // 本地地址
connection.originator_port = 0xA1B2; // 本地端口号
connection.target_address = inet_addr(ip_address); // 目标地址
connection.target_port = htons(port); // 目标端口号
connection.timeout_in_ticks = 1000;
// 打开连接
CipsterApi::OpenConnection(&connection);
// 构造 CIP 报文
CipByte message[1024];
CipByte* p = message;
// 先写入头部信息
*p++ = 0x01; // Command: SendRRData
*p++ = 0x00; // Reserved
*p++ = 0x00; // Length (高位)
*p++ = 0x00; // Length (低位)
// 写入请求路径
*p++ = 0x20; // Class ID: Message Router
*p++ = 0x06; // Instance ID: 6
*p++ = 0x24; // Attribute ID: Service
*p++ = 0x01; // Service Code: Multiple Service Packet
// 写入数据
*p++ = 0x0E; // 类型码: 8-bit Integer
*p++ = 0x02; // 数量: 2
*p++ = 0x10; // 数据: 16
*p++ = 0x20; // 数据: 32
// 计算报文长度
CipWord message_length = static_cast<CipWord>(p - message) - 4;
message[2] = static_cast<CipByte>(message_length >> 8);
message[3] = static_cast<CipByte>(message_length & 0xFF);
// 发送报文
CipByte response[1024];
CipsterApi::SendRequest(
&connection,
message,
message_length + 4,
response,
sizeof(response),
1000
);
// 解析响应报文
CipByte* q = response;
CipByte code = *q++;
CipByte service = *q++;
CipWord length = (*q++) << 8 | (*q++);
CipByte* data = q;
if (code != 0x02 || service != 0xCC) {
cerr << "Error: Invalid response" << endl;
return 1;
}
CipWord status = (*data++) << 8 | (*data++);
if (status != 0) {
cerr << "Error: Status code = " << status << endl;
return 1;
}
// 关闭连接
CipsterApi::CloseConnection(&connection);
return 0;
}
```
以上代码演示了如何使用 EntherNet/IP CIP 协议批量写入两个 8-bit 整数。在实际使用中,你需要根据自己的应用场景和设备配置进行相应的修改。同时,你需要了解 EntherNet/IP CIP 协议的具体规范和格式,以确保代码的正确性。
阅读全文
相关推荐













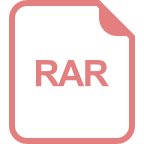




