如何将Excel表格中的薪资和学历之间关系绘制成饼图用matplotlib
时间: 2023-12-10 20:40:25 浏览: 101
首先,需要将 Excel 表格中的数据读取到 Python 中。可以使用 pandas 库来读取 Excel 表格中的数据。假设表格中有两列数据,分别为薪资和学历,可以使用以下代码读取数据:
```python
import pandas as pd
# 读取 Excel 表格中的数据
data = pd.read_excel('data.xlsx')
# 获取薪资和学历列的数据
salary = data['薪资']
education = data['学历']
```
接下来,需要统计每个学历对应的薪资总和。可以使用字典来保存每个学历的薪资总和,然后使用 matplotlib 库来绘制饼图。以下是完整代码示例:
```python
import pandas as pd
import matplotlib.pyplot as plt
# 读取 Excel 表格中的数据
data = pd.read_excel('data.xlsx')
# 获取薪资和学历列的数据
salary = data['薪资']
education = data['学历']
# 统计每个学历对应的薪资总和
salary_dict = {}
for i in range(len(salary)):
if education[i] not in salary_dict:
salary_dict[education[i]] = 0
salary_dict[education[i]] += salary[i]
# 绘制饼图
labels = salary_dict.keys()
sizes = salary_dict.values()
plt.pie(sizes, labels=labels, autopct='%1.1f%%')
plt.axis('equal')
plt.show()
```
这段代码会生成一个饼图,显示不同学历对应的薪资总和占比。其中,labels 变量保存了每个学历的名称,sizes 变量保存了每个学历对应的薪资总和。autopct 参数用来设置饼图上的百分比显示格式。最后,使用 plt.show() 函数显示饼图。
相关推荐
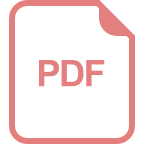
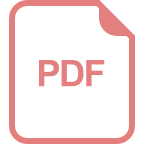














