public static void main(String[] args) { String url = ""; // 调用获取下载信息接口获取的internalResourceUrls。 String path = "/Users/xxxxx/Downloads/test.txt"; // 文件要下载的目标路径。 // 调用获取下载信息接口得到的headers信息。 Map<String, String> headers = "" //调用下载信息接口获取的headers。 OkHttpClient client = new OkHttpClient(); Request request = new Request.Builder() .url(url) .headers(Headers.of(headers)) .build(); client.newCall(request).enqueue(new Callback() { @Override public void onFailure(Call call, IOException e) { } @Override public void onResponse(Call call, Response response) throws IOException { Sink sink = null; BufferedSink bufferedSink = null; try { File dest = new File(path); sink = Okio.sink(dest); bufferedSink = Okio.buffer(sink); bufferedSink.writeAll(response.body().source()); } catch (Exception e) { e.printStackTrace(); } finally { if (bufferedSink != null) { bufferedSink.close(); } if (sink != null) { sink.close(); } } } }); }轉化為C#
时间: 2024-02-10 11:11:22 浏览: 114
好的,以下是将Java代码转换为C#代码的结果:
```csharp
static void Main(string[] args)
{
string url = ""; // 调用获取下载信息接口获取的internalResourceUrls。
string path = "/Users/xxxxx/Downloads/test.txt"; // 文件要下载的目标路径。
// 调用获取下载信息接口得到的headers信息。
Dictionary<string, string> headers = new Dictionary<string, string>(); // 调用下载信息接口获取的headers。
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.Url(url)
.Headers(Headers.Of(headers))
.Build();
client.NewCall(request).Enqueue(new CallbackAnonymousInnerClass());
}
private class CallbackAnonymousInnerClass : Callback
{
public void OnFailure(Call call, IOException e)
{
// 异常处理
}
public void OnResponse(Call call, Response response)
{
Sink sink = null;
BufferedSink bufferedSink = null;
try
{
FileInfo dest = new FileInfo(path);
sink = Okio.Sink(dest);
bufferedSink = Okio.Buffer(sink);
bufferedSink.WriteAll(response.Body.Source());
}
catch (Exception e)
{
e.PrintStackTrace();
}
finally
{
if (bufferedSink != null)
{
bufferedSink.Close();
}
if (sink != null)
{
sink.Close();
}
}
}
}
```
需要注意的是,由于C#和Java在语法上存在一些差异,所以在转换过程中需要进行一些微调。例如,Java中的Map类型需要转换为C#中的Dictionary类型。同时,C#中没有类似Java中的匿名内部类,因此需要手动创建一个实现了Callback接口的类。
阅读全文
相关推荐
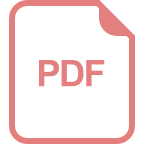
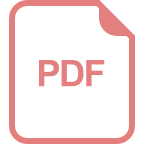
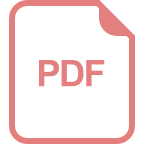
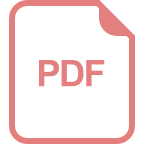














