判断A,B,C三个数大小,并将其从小到大代入x,y,z中,并且判断其中两个数是否能被3或7整除
时间: 2024-10-07 18:05:21 浏览: 26
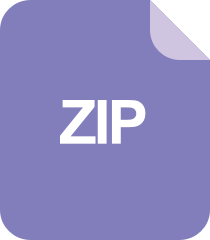
c代码-输入三个整数x,y,z,请把这三个数由小到大输出。

你可以使用C++来实现这个功能。首先,你需要比较三个数A、B、C的大小并确定它们分别对应变量x、y、z。然后检查每个数是否能被3或7整除。以下是一个简单的示例代码:
```cpp
#include <iostream>
bool isDivisible(int num, int divisor) {
return num % divisor == 0;
}
int main() {
int A, B, C;
std::cout << "Enter three numbers (A, B, C): ";
std::cin >> A >> B >> C;
if (A <= B && A <= C) {
x = A; // Assign the smallest number to x
y = B;
z = C;
} else if (B <= A && B <= C) {
x = B; // Assign the smallest number to x
y = A;
z = C;
} else {
x = C; // Assign the smallest number to x
y = A;
z = B;
}
bool divisibleBy3_x = isDivisible(x, 3);
bool divisibleBy3_y = isDivisible(y, 3);
bool divisibleBy3_z = isDivisible(z, 3);
bool divisibleBy7_x = isDivisible(x, 7);
bool divisibleBy7_y = isDivisible(y, 7);
bool divisibleBy7_z = isDivisible(z, 7);
std::cout << "Numbers ordered as x=" << x << ", y=" << y << ", z=" << z << "\n";
std::cout << "x can be divided by 3: " << divisibleBy3_x << ", by 7: " << divisibleBy7_x << "\n";
std::cout << "y can be divided by 3: " << divisibleBy3_y << ", by 7: " << divisibleBy7_y << "\n";
std cout << "z can be divided by 3: " << divisibleBy3_z << ", by 7: " << divisibleBy7_z << "\n";
return 0;
}
```
在这个代码中,`isDivisible` 函数用来检查一个数是否能被指定的数字整除。主函数里先获取三个数,然后根据条件排序,并检查每个排序后的数是否能被3或7整除。
阅读全文
相关推荐
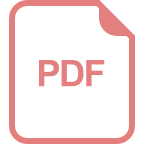
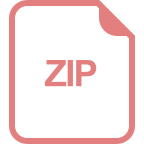
















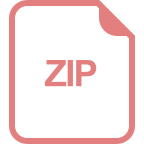