用c语言写一个五子棋机器人
时间: 2024-05-09 19:15:50 浏览: 97
好的,我会给你提供一个基本的五子棋机器人的代码框架,但需要注意的是,这只是一个基本的框架,需要根据实际情况进行修改和完善。
```
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define SIZE 15
#define PLAYER 1
#define COMPUTER 2
#define EMPTY 0
int chessboard[SIZE][SIZE]; // 棋盘数组
int color[2] = {1, 2}; // 玩家和电脑的颜色
int current_color; // 当前落子的颜色
// 初始化棋盘
void init_chessboard()
{
int i, j;
for(i = 0; i < SIZE; i++) {
for(j = 0; j < SIZE; j++) {
chessboard[i][j] = EMPTY;
}
}
}
// 打印棋盘
void print_chessboard()
{
int i, j;
for(i = 0; i < SIZE; i++) {
for(j = 0; j < SIZE; j++) {
printf("%d ", chessboard[i][j]);
}
printf("\n");
}
}
// 判断当前位置是否能落子
int is_valid_position(int x, int y)
{
if(x < 0 || x >= SIZE || y < 0 || y >= SIZE) {
return 0;
}
if(chessboard[x][y] != EMPTY) {
return 0;
}
return 1;
}
// 玩家落子
void player_move()
{
int x, y;
printf("请输入落子位置的坐标(x,y):");
scanf("%d,%d", &x, &y);
while(!is_valid_position(x, y)) {
printf("输入错误,请重新输入:");
scanf("%d,%d", &x, &y);
}
chessboard[x][y] = PLAYER;
}
// 电脑落子
void computer_move()
{
int x, y;
// TODO: 编写电脑落子的算法
// 在这里使用随机算法作为示例
srand(time(NULL));
do {
x = rand() % SIZE;
y = rand() % SIZE;
} while(!is_valid_position(x, y));
chessboard[x][y] = COMPUTER;
}
// 判断是否有五子连珠
int check_win(int x, int y)
{
int i, j;
int count;
int current = chessboard[x][y];
// 判断横向
count = 1;
for(i = x - 1; i >= 0 && chessboard[i][y] == current; i--) {
count++;
}
for(i = x + 1; i < SIZE && chessboard[i][y] == current; i++) {
count++;
}
if(count >= 5) {
return current;
}
// 判断纵向
count = 1;
for(j = y - 1; j >= 0 && chessboard[x][j] == current; j--) {
count++;
}
for(j = y + 1; j < SIZE && chessboard[x][j] == current; j++) {
count++;
}
if(count >= 5) {
return current;
}
// 判断左上到右下斜线
count = 1;
for(i = x - 1, j = y - 1; i >= 0 && j >= 0 && chessboard[i][j] == current; i--, j--) {
count++;
}
for(i = x + 1, j = y + 1; i < SIZE && j < SIZE && chessboard[i][j] == current; i++, j++) {
count++;
}
if(count >= 5) {
return current;
}
// 判断左下到右上斜线
count = 1;
for(i = x - 1, j = y + 1; i >= 0 && j < SIZE && chessboard[i][j] == current; i--, j++) {
count++;
}
for(i = x + 1, j = y - 1; i < SIZE && j >= 0 && chessboard[i][j] == current; i++, j--) {
count++;
}
if(count >= 5) {
return current;
}
return EMPTY;
}
// 判断是否平局
int check_draw()
{
int i, j;
for(i = 0; i < SIZE; i++) {
for(j = 0; j < SIZE; j++) {
if(chessboard[i][j] == EMPTY) {
return 0;
}
}
}
return 1;
}
// 主函数
int main()
{
int winner = EMPTY;
init_chessboard();
print_chessboard();
current_color = PLAYER;
while(winner == EMPTY && !check_draw()) {
if(current_color == PLAYER) {
player_move();
current_color = COMPUTER;
} else {
computer_move();
current_color = PLAYER;
}
print_chessboard();
winner = check_win(x, y);
}
if(winner == PLAYER) {
printf("你赢了!\n");
} else if(winner == COMPUTER) {
printf("电脑赢了!\n");
} else {
printf("平局!\n");
}
return 0;
}
```
上面的代码是一个基本的五子棋机器人的框架,其中有些函数需要你自己根据实际情况进行修改和完善,比如`computer_move()`函数中的电脑落子算法。你可以使用各种算法来实现电脑的落子,比如随机算法、极大极小值算法、Alpha-Beta剪枝算法等。
阅读全文
相关推荐
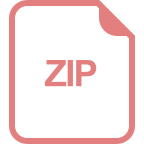
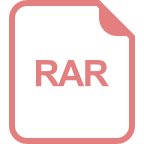
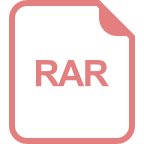
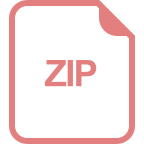
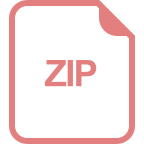
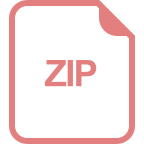
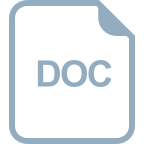
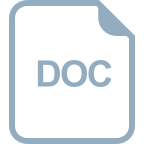
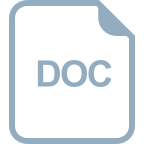




