编写程序,从给定字符串中查找某指定的字符
时间: 2023-05-31 20:18:25 浏览: 572
### 回答1:
可以使用字符串的find()方法来查找指定字符在字符串中的位置。例如,假设要查找字符'c'在字符串s中的位置,可以使用以下代码:
```
s = "hello world"
pos = s.find('c')
if pos == -1:
print("未找到指定字符")
else:
print("指定字符在字符串中的位置为:", pos)
```
输出结果为:
```
未找到指定字符
```
说明在字符串中没有找到字符'c'。如果要查找字符'l'在字符串s中的位置,可以将代码中的'c'改为'l',输出结果为:
```
指定字符在字符串中的位置为: 2
```
说明字符'l'在字符串s中的位置为2。
### 回答2:
查找某指定字符是程序设计中非常常见的操作,而且对于许多应用也非常必要。下面介绍一种简单的方法,可以用来从给定字符串中查找某指定的字符:
首先,程序应该要求用户输入要查找的字符串以及要查找的字符。这可以使用标准输入函数来实现。
接下来,程序应该遍历整个字符串,找到每个字符,并与用户指定的字符进行比较。如果找到了指定的字符,程序应该将该字符的位置记录下来,并继续查找,直到遍历完字符串。
最后,程序应该输出所有找到的指定字符出现的位置。如果没有找到,程序应该输出相应信息,提示用户。
以下是示例代码:
#include <stdio.h>
#include <string.h>
int main() {
char str[1000], c;
int i, len, index[100], count = 0;
// 读取字符串及要查找的字符
printf("请输入字符串:");
fgets(str, sizeof(str), stdin);
printf("请输入要查找的字符:");
scanf("%c", &c);
len = strlen(str);
// 查找字符
for (i = 0; i < len; i++) {
if (str[i] == c) {
index[count] = i;
count++;
}
}
// 输出查找结果
if (count == 0) {
printf("在字符串中没有找到指定字符。\n");
} else {
printf("在字符串中找到了%d个指定字符,它们的位置分别为:", count);
for (i = 0; i < count; i++) {
printf("%d ", index[i]);
}
printf("\n");
}
return 0;
}
在上面的程序中,我们首先读取了用户输入的字符串和要查找的字符。然后,在 for 循环中遍历整个字符串,查找用户指定的字符。如果找到了指定字符,则将其位置记录下来。最后,我们输出查找结果。如果没有找到,我们会输出相应的提示信息。
这段程序较短、易懂,适合初学者用来学习字符串处理和字符查找等基本内容。如果要处理更为复杂的问题,可以在此基础上进行扩展和改进。
### 回答3:
编写程序,从给定字符串中查找某指定的字符,可以采用以下几种方法:
1.使用string类的find函数:string类中的find函数可以用来查找字符串中某个字符的位置,函数原型如下:size_t find(const char *str, size_t pos = 0) const;其中,str表示要查找的字符,pos表示从字符串的哪个位置开始查找,默认值为0。该函数返回查找到的第一个字符的位置,如果没有找到,则返回string::npos。示例代码如下:
```
#include <iostream>
#include <string>
using namespace std;
int main()
{
string s = "hello world";
char c = 'o';
size_t pos = s.find(c);
if(pos == string::npos)
{
cout << "未找到字符" << endl;
}
else
{
cout << "字符" << c << "在字符串中的位置为:" << pos << endl;
}
return 0;
}
```
2.使用STL中的algorithms库函数:STL中的algorithms库函数提供了一系列的查找算法,其中包括find函数和count函数,这两个函数都可以用来查找字符。示例代码如下:
```
#include <iostream>
#include <algorithm>
#include <string>
using namespace std;
int main()
{
string s = "hello world";
char c = 'o';
string::iterator it = find(s.begin(), s.end(), c);
if(it == s.end())
{
cout << "未找到字符" << endl;
}
else
{
cout << "字符" << c << "在字符串中的位置为:" << it-s.begin() << endl;
}
return 0;
}
```
3.使用普通的for循环遍历:通过for循环遍历字符串,逐个查找指定字符,如果找到了,则返回其位置,如果遍历完整个字符串都没有找到,则返回一个特定的值(如-1)。示例代码如下:
```
#include <iostream>
#include <cstring>
using namespace std;
int main()
{
char s[] = "hello world";
char c = 'o';
int pos = -1;
for(int i=0; i<strlen(s); i++)
{
if(s[i] == c)
{
pos = i;
break;
}
}
if(pos == -1)
{
cout << "未找到字符" << endl;
}
else
{
cout << "字符" << c << "在字符串中的位置为:" << pos << endl;
}
return 0;
}
```
以上三种方法都是常用的从给定字符串中查找某指定的字符的方法,可以根据实际需求选择其中的一种或几种方法。
相关推荐
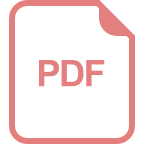
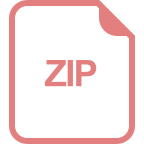
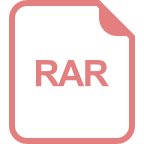














