请给出具体的车牌校正程序(python3.9,opencv4.7)
时间: 2024-02-17 10:00:51 浏览: 62
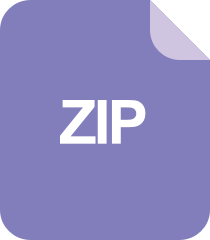
利用Python opencv进行车牌识别

以下是基于Python3.9和OpenCV4.7的车牌定位与畸变校正的完整实现代码:
```python
import cv2
import numpy as np
# 车牌定位
def locate_license_plate(img):
# 高斯滤波
img = cv2.GaussianBlur(img, (3, 3), 0)
# Sobel算子
sobel = cv2.Sobel(img, cv2.CV_8U, 1, 0, ksize=3)
# 二值化
ret, binary = cv2.threshold(sobel, 0, 255, cv2.THRESH_OTSU+cv2.THRESH_BINARY)
# 闭运算
kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (17, 5))
closed = cv2.morphologyEx(binary, cv2.MORPH_CLOSE, kernel)
# 轮廓检测
contours, hierarchy = cv2.findContours(closed, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_NONE)
# 筛选轮廓
max_area = 0
best_cnt = None
for cnt in contours:
area = cv2.contourArea(cnt)
if area < 2000 or area > 20000:
continue
peri = cv2.arcLength(cnt, True)
approx = cv2.approxPolyDP(cnt, 0.02 * peri, True)
if len(approx) != 4:
continue
if area > max_area:
max_area = area
best_cnt = approx
# 提取车牌轮廓
if best_cnt is not None:
x, y, w, h = cv2.boundingRect(best_cnt)
license_plate = img[y:y+h, x:x+w]
return license_plate
else:
return None
# 畸变校正
def correct_perspective(license_plate_img):
# 灰度化
gray = cv2.cvtColor(license_plate_img, cv2.COLOR_BGR2GRAY)
# 二值化
ret, binary = cv2.threshold(gray, 0, 255, cv2.THRESH_OTSU+cv2.THRESH_BINARY)
# 查找轮廓
contours, hierarchy = cv2.findContours(binary, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 找到最大轮廓
max_area = 0
best_cnt = None
for cnt in contours:
area = cv2.contourArea(cnt)
if area < 1000 or area > 10000:
continue
peri = cv2.arcLength(cnt, True)
approx = cv2.approxPolyDP(cnt, 0.02 * peri, True)
if len(approx) != 4:
continue
if area > max_area:
max_area = area
best_cnt = approx
# 找到四个角点
rect = cv2.minAreaRect(best_cnt)
box = cv2.boxPoints(rect)
box = np.int0(box)
# 计算变换矩阵
width = int(np.sqrt((box[0][0]-box[1][0])**2+(box[0][1]-box[1][1])**2))
height = int(np.sqrt((box[0][0]-box[3][0])**2+(box[0][1]-box[3][1])**2))
src_pts = box.astype("float32")
dst_pts = np.array([[0, 0], [width-1, 0], [width-1, height-1], [0, height-1]], dtype="float32")
M = cv2.getPerspectiveTransform(src_pts, dst_pts)
# 变换
warped = cv2.warpPerspective(license_plate_img, M, (width, height))
return warped
# 主函数
if __name__ == '__main__':
# 读取图像
img = cv2.imread('image.jpg')
# 车牌定位
license_plate_img = locate_license_plate(img)
# 畸变校正
corrected_license_plate_img = correct_perspective(license_plate_img)
# 显示结果
cv2.imshow('original image', img)
cv2.imshow('license plate', license_plate_img)
cv2.imshow('corrected license plate', corrected_license_plate_img)
cv2.waitKey()
```
其中,`locate_license_plate`函数实现了车牌定位,`correct_perspective`函数实现了畸变校正。主函数读取图像,进行车牌定位和畸变校正,并显示结果。
阅读全文
相关推荐
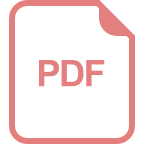
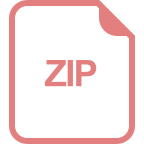
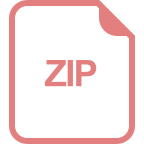
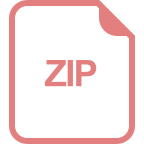
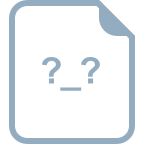
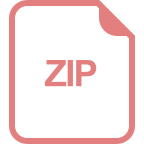
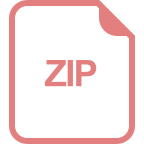
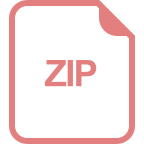
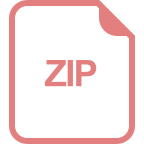
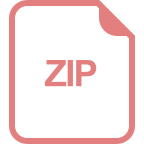
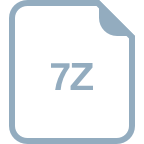
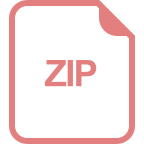
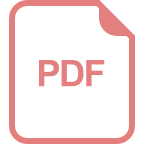
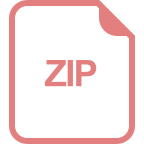
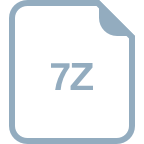
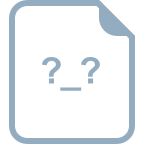