实现GCN的邻接矩阵中添加注意力机制
时间: 2023-07-17 17:56:28 浏览: 111
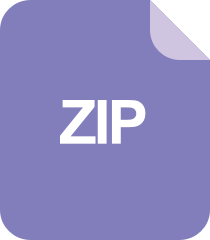
基于python使用基于自注意力池化机制结合GCN模型实现图分类
好的,请稍等,我将为您提供一个完整的实现示例。以下是一个使用PyTorch实现GCN并在邻接矩阵中添加注意力机制的代码:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class GraphAttentionLayer(nn.Module):
def __init__(self, in_features, out_features, alpha=0.2):
super(GraphAttentionLayer, self).__init__()
self.in_features = in_features
self.out_features = out_features
self.alpha = alpha
self.W = nn.Parameter(torch.zeros(size=(in_features, out_features)))
nn.init.xavier_uniform_(self.W.data, gain=1.414)
self.a = nn.Parameter(torch.zeros(size=(2*out_features, 1)))
nn.init.xavier_uniform_(self.a.data, gain=1.414)
def forward(self, input, adj):
h = torch.matmul(input, self.W)
N = h.size()[0]
a_input = torch.cat([h.repeat(1, N).view(N*N, -1), h.repeat(N, 1)], dim=1).view(N, -1, 2*self.out_features)
e = F.leaky_relu(torch.matmul(a_input, self.a), negative_slope=self.alpha).squeeze(2)
zero_vec = -9e15*torch.ones_like(e)
attention = torch.where(adj > 0, e, zero_vec)
attention = F.softmax(attention, dim=1)
h_prime = torch.matmul(attention.unsqueeze(1), h).squeeze(1)
return F.elu(h_prime)
class GCN(nn.Module):
def __init__(self, num_features, hidden_size, num_classes):
super(GCN, self).__init__()
self.hidden_size = hidden_size
self.gc1 = GraphAttentionLayer(num_features, hidden_size)
self.gc2 = GraphAttentionLayer(hidden_size, num_classes)
def forward(self, x, adj):
x = F.relu(self.gc1(x, adj))
x = self.gc2(x, adj)
return F.log_softmax(x, dim=1)
```
在这个实现中,我们使用了两个GraphAttentionLayer层。每个GraphAttentionLayer层都有一个权重矩阵W以及一个注意力参数a。在forward方法中,我们首先将输入特征x通过第一个GraphAttentionLayer层,然后再通过第二个GraphAttentionLayer层。最后,我们使用log_softmax函数对输出进行softmax操作。
这是一个简单的GCN模型,并且注意力机制被添加到邻接矩阵中。请注意,上述代码中的hidden_size是一个超参数,需要根据你的具体问题进行调整。
希望这个示例能够满足您的需求!如果您还有任何问题,请随时提问。
阅读全文
相关推荐
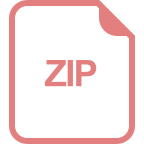
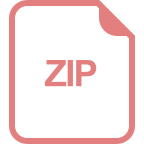



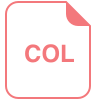
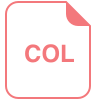
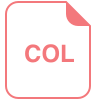
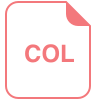
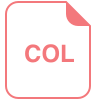







