在GCN的邻接矩阵中添加注意力机制 python
时间: 2023-07-17 09:56:21 浏览: 125
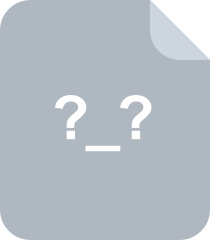
注意力机制代码 python
要在GCN的邻接矩阵中添加注意力机制,你可以使用以下步骤:
1. 导入所需的库:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
```
2. 创建一个注意力机制的类:
```python
class GraphAttentionLayer(nn.Module):
def __init__(self, in_features, out_features):
super(GraphAttentionLayer, self).__init__()
self.in_features = in_features
self.out_features = out_features
self.W = nn.Parameter(torch.zeros(size=(in_features, out_features)))
nn.init.xavier_uniform_(self.W.data, gain=1.414)
def forward(self, input, adj):
h = torch.matmul(input, self.W)
N = h.size()[0]
a_input = torch.cat([h.repeat(1, N).view(N * N, -1), h.repeat(N, 1)], dim=1).view(N, -1, 2 * self.out_features)
e = F.leaky_relu(torch.matmul(a_input, self.a), negative_slope=0.2)
zero_vec = -9e15*torch.ones_like(e)
attention = torch.where(adj > 0, e, zero_vec)
attention = F.softmax(attention, dim=1)
h_prime = torch.matmul(attention, h)
return h_prime
```
3. 创建一个GCN模型类,并在其中使用注意力机制:
```python
class GCN(nn.Module):
def __init__(self, num_features, num_classes):
super(GCN, self).__init__()
self.layer1 = GraphAttentionLayer(num_features, hidden_size)
self.layer2 = GraphAttentionLayer(hidden_size, num_classes)
def forward(self, x, adj):
x = F.relu(self.layer1(x, adj))
x = self.layer2(x, adj)
return x
```
这是一个简单的GCN模型,其中的GraphAttentionLayer类实现了注意力机制。在forward方法中,我们首先将输入特征x通过第一个注意力层,然后再通过第二个注意力层。最后,返回输出结果。
请注意,上述代码中的hidden_size是一个超参数,需要根据你的具体问题进行调整。另外,还需要定义一个邻接矩阵adj作为输入,这可以表示图中节点之间的连接关系。
希望这可以帮助你添加注意力机制到GCN中!如果你有任何进一步的问题,请随时提问。
阅读全文
相关推荐
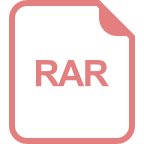


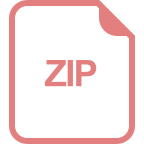
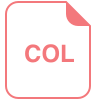











