python使用tensorflow库实现GCN模型,将邻接矩阵和特征矩阵输入模型中进行嵌入。
时间: 2024-03-05 14:54:08 浏览: 170
好的,您的问题是如何使用Python中的TensorFlow库实现GCN模型,并将邻接矩阵和特征矩阵输入模型中进行嵌入。
GCN(Graph Convolutional Network)是一种深度学习模型,用于处理图数据。它是通过在图上执行卷积操作来处理节点特征的一种方法。TensorFlow是一种广泛使用的深度学习框架,提供了许多工具和函数来简化构建和训练深度学习模型的过程。
以下是使用TensorFlow实现GCN模型的步骤:
1.导入所需的库和模块:
```python
import tensorflow as tf
import numpy as np
```
2.定义GCN模型的参数:
```python
num_nodes = 100 # 图的节点数
input_dim = 50 # 特征矩阵的维度
hidden_dim = 64 # 隐藏层的维度
output_dim = 2 # 输出的维度
learning_rate = 0.01 # 学习率
```
3.定义邻接矩阵和特征矩阵:
```python
adjacency_matrix = tf.placeholder(tf.float32, shape=[num_nodes, num_nodes])
feature_matrix = tf.placeholder(tf.float32, shape=[num_nodes, input_dim])
```
4.定义模型的权重和偏差:
```python
weights = {
'hidden': tf.Variable(tf.random_normal([input_dim, hidden_dim])),
'output': tf.Variable(tf.random_normal([hidden_dim, output_dim]))
}
biases = {
'hidden': tf.Variable(tf.random_normal([hidden_dim])),
'output': tf.Variable(tf.random_normal([output_dim]))
}
```
5.定义GCN模型的前向传播过程:
```python
hidden_layer = tf.nn.relu(tf.matmul(tf.matmul(adjacency_matrix, feature_matrix), weights['hidden']) + biases['hidden'])
output_layer = tf.matmul(hidden_layer, weights['output']) + biases['output']
```
6.定义损失函数和优化器:
```python
labels = tf.placeholder(tf.float32, shape=[num_nodes, output_dim])
loss = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits_v2(logits=output_layer, labels=labels))
optimizer = tf.train.AdamOptimizer(learning_rate=learning_rate).minimize(loss)
```
7.训练模型:
```python
with tf.Session() as sess:
sess.run(tf.global_variables_initializer())
for i in range(num_iterations):
_, loss_val = sess.run([optimizer, loss], feed_dict={adjacency_matrix: adj, feature_matrix: features, labels: labels})
if i % 10 == 0:
print("Iteration:", i, "Loss:", loss_val)
```
其中,`adj`、`features`和`labels`是预处理过的邻接矩阵、特征矩阵和标签矩阵。
这就是使用Python中的TensorFlow库实现GCN模型,并将邻接矩阵和特征矩阵输入模型中进行嵌入的方法。
阅读全文
相关推荐
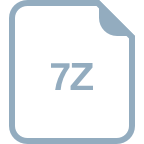
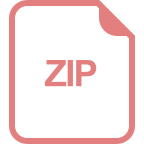
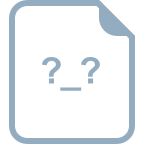


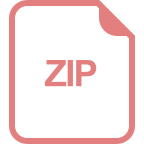
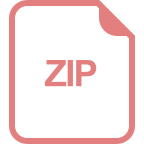
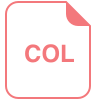










