c语言已经启动了多个线程(1 2 3)时,主线程需要延时2s,怎么在主线程延时的同时不影响所有子线程,子线程仍正常运行
时间: 2024-09-27 19:04:06 浏览: 33
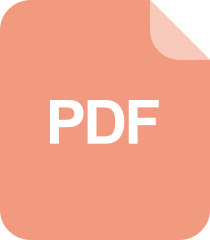
延时函数-python matplotlib 画dataframe的时间序列图实例
在C语言中,为了在主线程延迟期间让子线程不受影响地继续执行,你可以使用互斥锁(mutex)或者条件变量(condition variable)。下面是一个简单的例子,利用`pthread_mutex_t`和`pthread_cond_t`来实现:
```c
#include <pthread.h>
#include <time.h>
#define THREADS 3
#define DELAY_TIME 2
// 全局互斥锁和信号量
pthread_mutex_t mutex;
pthread_cond_t cond;
void* thread_func(void *thread_id) {
// 线程函数,获取锁并开始工作
pthread_mutex_lock(&mutex);
printf("Thread %ld started\n", (long)thread_id);
// 在这里做你的任务...
// 工作完成后,释放锁并通知主线程延迟结束
pthread_mutex_unlock(&mutex);
pthread_cond_signal(&cond);
}
int main() {
pthread_t threads[THREADS];
int i;
// 初始化互斥锁和信号量
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(&cond, NULL);
// 创建子线程
for (i = 0; i < THREADS; ++i) {
pthread_create(&threads[i], NULL, thread_func, (void*)i);
}
// 主线程延时
printf("Main thread delaying...\n");
sleep(DELAY_TIME * 1000); // 调整单位以便秒级计时
// 解锁并唤醒所有等待的线程
pthread_mutex_lock(&mutex);
pthread_cond_broadcast(&cond);
pthread_mutex_unlock(&mutex);
// 等待所有线程完成
for (i = 0; i < THREADS; ++i) {
pthread_join(threads[i], NULL);
}
// 销毁互斥锁和信号量
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(&cond);
return 0;
}
```
在这个示例中,主线程先创建子线程,然后进入睡眠状态。当主线程醒来时,它会先锁定互斥锁,然后广播信号给其他等待的线程,让它们继续执行。这种方式保证了主线程的延时不会阻塞子线程。
阅读全文
相关推荐
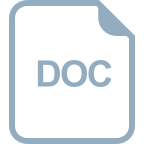
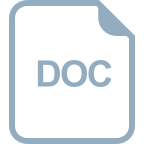


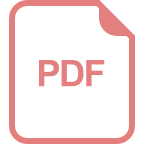
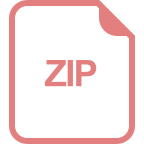
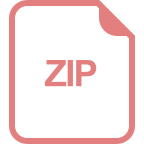
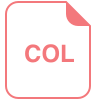









