C#WMI在远程主机上的运行远程主机上的程序
时间: 2024-10-24 13:09:07 浏览: 28
C#中的WMI(Windows Management Instrumentation)是一种用于管理系统信息和执行系统管理任务的技术。通过WMI,你可以在远程主机上运行程序。以下是一些关键步骤和示例代码来演示如何使用C#和WMI在远程主机上运行程序:
### 步骤:
1. **添加引用**:在你的C#项目中,需要添加对`System.Management`的引用。
2. **连接远程计算机**:使用`ManagementScope`类连接到远程计算机。
3. **创建并执行WMI方法**:使用`ManagementClass`和`InvokeMethod`方法在远程计算机上执行程序。
### 示例代码:
```csharp
using System;
using System.Management;
class Program
{
static void Main()
{
try
{
// 设置远程计算机的名称或IP地址
string remoteComputer = "RemoteComputerNameOrIP";
// 创建一个连接到远程计算机的连接选项
ConnectionOptions options = new ConnectionOptions
{
Username = "username",
Password = "password",
Impersonation = ImpersonationLevel.Impersonate,
Authentication = AuthenticationLevel.PacketPrivacy
};
// 初始化ManagementScope对象,连接到远程计算机
ManagementScope scope = new ManagementScope(@"\\" + remoteComputer + @"\root\cimv2", options);
scope.Connect();
// 创建一个对象,用于调用WMI方法
ManagementClass wmiClass = new ManagementClass(scope, new ManagementPath("Win32_Process"), null);
// 定义要启动的程序的路径和参数
string programPath = @"C:\Path\To\YourProgram.exe";
string[] args = { "arg1", "arg2" }; // 如果程序有命令行参数,可以在这里指定
// 调用WMI方法,启动远程进程
ManagementBaseObject inParams = wmiClass.GetMethodParameters("Create");
inParams["CommandLine"] = programPath + " " + string.Join(" ", args);
ManagementBaseObject outParams = wmiClass.InvokeMethod("Create", inParams, null);
Console.WriteLine("Program executed successfully on the remote computer!");
}
catch (Exception ex)
{
Console.WriteLine("An error occurred: " + ex.Message);
}
}
}
```
### 注意事项:
- 确保远程计算机上的防火墙允许WMI流量。
- 你需要提供有效的用户名和密码以进行身份验证。
- 目标程序的路径必须是绝对路径。
阅读全文
相关推荐
















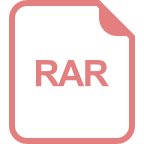
