C#使用WMI启动在远程主机上运行的程序
时间: 2024-10-24 20:16:35 浏览: 15
在C#中,你可以使用Windows Management Instrumentation (WMI) 来执行远程主机上的命令,但这通常涉及权限管理和网络安全限制。以下是一个简单的示例,展示如何使用`ManagementScope`和`ManagementJob`类来在远程机器上执行命令:
```csharp
using System;
using System.Management;
using System.Management.Automation;
public class RemoteExecution
{
public static void Main(string[] args)
{
// 假设你有远程机器的IP地址和凭据
string remoteMachine = "192.168.1.1";
string username = "domain\\username";
string password = "password";
// 创建连接到远程主机的管理范围
using (var scope = new ManagementScope("\\\\" + remoteMachine + "\\root\\cimv2",
new ManagementPath("Win32_Process"),
new AuthenticationProvider(username, password))
)
{
// 使用PowerShell脚本执行远程命令
string script = @"& 'C:\path\to\your\script.exe'"; // 替换为你想执行的文件路径
ManagementJob job = null;
try
{
// 创建并启动一个新的作业
job = scope.CreateJob();
ManagementBaseObject inParams = job.GetParameters()[0];
inParams.PutValue(script);
job.Run();
Console.WriteLine("Job started on remote machine with ID: " + job.JobId);
}
catch (Exception ex)
{
Console.WriteLine("Error executing command: " + ex.Message);
}
// 监听作业状态直到完成(可选)
while (job.Status != JobStatus.Completed && job.Status != JobStatus.Failed)
{
Thread.Sleep(1000); // 每秒检查一次
Console.Write(".");
}
if (job.Status == JobStatus.Completed)
{
Console.WriteLine("\nJob completed successfully.");
}
else if (job.Status == JobStatus.Failed)
{
Console.WriteLine("\nJob failed with error: " + job.GetResults().ToString());
}
}
}
}
```
注意:这个示例假设你的应用程序有足够的权限在远程主机上执行操作,并且网络环境允许跨机器通信。实际使用时可能需要考虑防火墙设置、安全策略以及认证验证等问题。
阅读全文
相关推荐
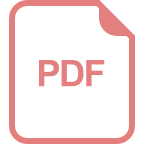
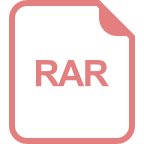
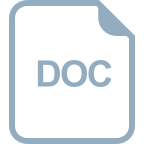











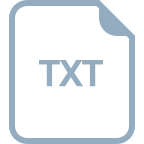
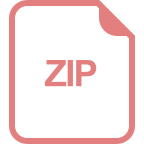
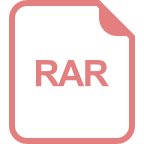
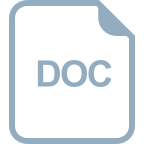