从自然数中取三个数进行组合之递归算法的伪代码
时间: 2024-09-28 10:13:57 浏览: 37
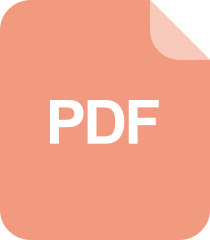
【算法设计与分析】期末汇总
当我们需要编写一个递归算法来从自然数序列中取出三个数的所有可能组合时,可以使用这种方法。这里是一个简单的伪代码示例:
```plaintext
function combination_of_three(n):
// 基线条件:当n小于3时,返回空列表,因为无法选取三个数
if n < 3:
return []
// 当前基本情况:取第一个数(0到n-1)
result = [ [n - 2, n - 1, n] ] // 例如,如果n=3,组合就是[0,1,2]
// 遍历剩余的n-1个数,将其与当前的第一个数组合
for i in range(0, n - 1):
// 取出剩下的两个数
sub_combinations = combination_of_three(i)
// 对每个子组合添加当前的第一个数作为新的一组
for sub_combination in sub_combinations:
new_combination = [i] + sub_combination
result.append(new_combination)
return result
阅读全文
相关推荐
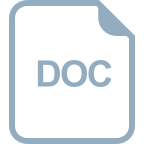
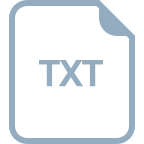

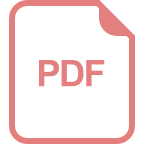
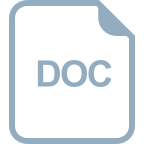
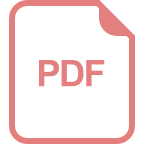
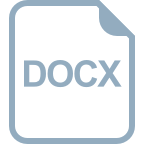
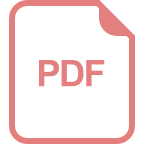
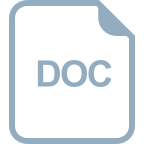
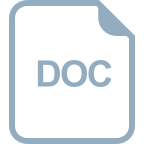
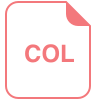
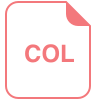
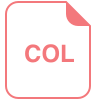
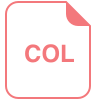
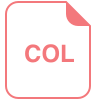

