在c语言中,分别使用递归和迭代两种方法编写二叉搜索树的查找函数
时间: 2024-10-21 20:15:17 浏览: 48
在C语言中,我们可以使用递归和迭代两种方法来实现二叉搜索树的查找功能。下面是两个版本的示例代码:
**递归方法**:
```c
typedef struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
TreeNode* searchBSTRecursively(TreeNode* root, int target) {
// 如果根节点为空或者等于目标值,直接返回
if (root == NULL || root->val == target) {
return root;
}
// 根据目标值与当前节点值的大小关系,递归查找左子树或右子树
if (target < root->val) {
return searchBSTRecursively(root->left, target);
} else {
return searchBSTRecursively(root->right, target);
}
}
```
**迭代方法**(使用栈实现):
```c
#include <stdbool.h>
#include <stdio.h>
bool searchBSTIteratively(TreeNode* root, int target) {
stack<TreeNode*> s;
TreeNode* current = root;
while (current != NULL || !s.isEmpty()) {
while (current != NULL) {
s.push(current);
current = current->val < target ? current->right : current->left;
}
current = s.pop();
if (current->val == target) {
return true;
}
current = current->val > target ? current->left : current->right;
}
return false;
}
// 这里假设你有一个辅助函数isEmpty()检查栈是否为空
```
在这两个示例中,递归方法通过不断地将子问题压入堆栈来查找,而迭代方法则是手动维护一个堆栈来存储待访问的节点。两者都可以有效地在二叉搜索树中查找指定的目标值。
阅读全文
相关推荐
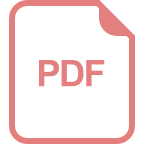
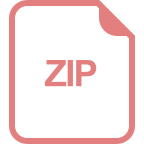
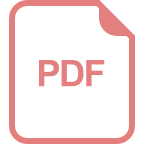
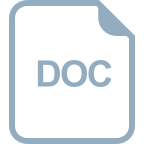
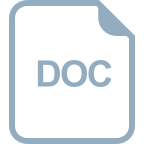
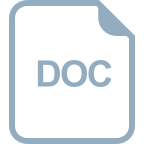
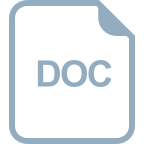
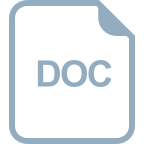
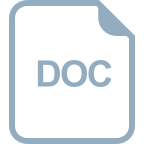
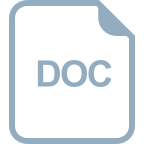
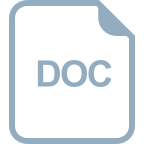
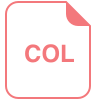
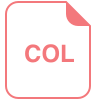
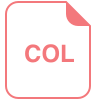
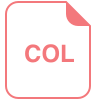
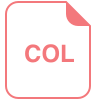
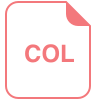

