用java解决以下问题,问题描述: 试完成一款桌面打字练习软件,能够测试人们的键盘输入能力。 要求: (1)测试文章以文本文件形式存放; (2)用户界面简单易操作 系统功能分析: (1)设置功能:可以选择文章,利用文件选择器JFileChooser选择并导入一篇文章; (2)打字输入功能;能够利用文本框接受用户输入, (3)统计功能:可以统计输入总字数、正确和错误字数,
时间: 2024-02-28 11:53:51 浏览: 17
以下是用Java解决这个问题的一个示例代码:
```java
import java.awt.BorderLayout;
import java.awt.EventQueue;
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JTextField;
public class TypingTest {
private JFrame frame;
private JFileChooser fileChooser;
private File textFile;
private BufferedReader reader;
private JPanel typingPanel;
private JTextField inputField;
private JLabel statsLabel;
private int totalChars;
private int correctChars;
private int wrongChars;
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
TypingTest window = new TypingTest();
window.frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
public TypingTest() {
initialize();
}
private void initialize() {
// Initialize the main window
frame = new JFrame();
frame.setBounds(100, 100, 500, 300);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.getContentPane().setLayout(new BorderLayout(0, 0));
// Initialize the file chooser
fileChooser = new JFileChooser();
// Initialize the typing panel
typingPanel = new JPanel();
typingPanel.setLayout(new FlowLayout(FlowLayout.CENTER, 10, 10));
frame.getContentPane().add(typingPanel, BorderLayout.CENTER);
// Initialize the input field
inputField = new JTextField();
inputField.setColumns(30);
inputField.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
processInput();
}
});
typingPanel.add(inputField);
// Initialize the stats label
statsLabel = new JLabel();
typingPanel.add(statsLabel);
// Initialize the button panel
JPanel buttonPanel = new JPanel();
buttonPanel.setLayout(new FlowLayout(FlowLayout.CENTER, 10, 10));
frame.getContentPane().add(buttonPanel, BorderLayout.SOUTH);
// Initialize the open file button
JButton openFileButton = new JButton("Open File");
openFileButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
openFile();
}
});
buttonPanel.add(openFileButton);
// Initialize the reset button
JButton resetButton = new JButton("Reset");
resetButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
resetStats();
}
});
buttonPanel.add(resetButton);
}
private void openFile() {
int result = fileChooser.showOpenDialog(frame);
if (result == JFileChooser.APPROVE_OPTION) {
textFile = fileChooser.getSelectedFile();
try {
reader = new BufferedReader(new FileReader(textFile));
inputField.setEnabled(true);
inputField.requestFocus();
resetStats();
displayStats();
} catch (IOException e) {
e.printStackTrace();
}
}
}
private void processInput() {
String input = inputField.getText().trim();
if (input.length() > 0) {
totalChars += input.length();
try {
String line = reader.readLine();
int i = 0;
while (line != null && i < input.length()) {
if (line.charAt(i) == input.charAt(i)) {
correctChars++;
} else {
wrongChars++;
}
i++;
}
if (line == null) {
inputField.setEnabled(false);
} else {
displayStats();
}
} catch (IOException e) {
e.printStackTrace();
}
inputField.setText("");
}
}
private void resetStats() {
totalChars = 0;
correctChars = 0;
wrongChars = 0;
inputField.setEnabled(false);
statsLabel.setText("");
}
private void displayStats() {
String stats = String.format("Total: %d Correct: %d Wrong: %d", totalChars, correctChars, wrongChars);
statsLabel.setText(stats);
}
}
```
这个程序使用了Swing框架来创建用户界面,包括一个主窗口和一个包含输入框和统计信息的面板。它还使用了JFileChooser来让用户选择要测试的文本文件。
当用户选择一个文本文件后,程序会读取文件并让输入框变为可用状态。用户可以在输入框中逐字输入文本,并且每次输入后程序会根据输入的正确性更新统计信息。当用户完成输入后,输入框会变为不可用状态,并且显示最终的统计信息。
在这个程序中,输入的正确性是根据用户输入的每个字符与文件中相应位置的字符进行比较来确定的。如果它们相等,就认为输入是正确的,否则就认为输入是错误的。
相关推荐
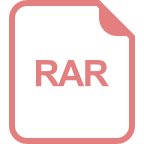
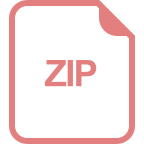
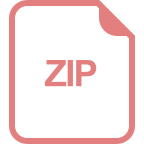















