Design a student class CStudent and complete the relevant content as required. Private data member: student name (stuName), student number (stuNum), math score (mathSco), physics score (phiSco); Constructor and Destructor; Public member function: myset() to modify grades, show() to display information; The friend function aver () is used to calculate the average score. Main function: two class objects “stu1” and “stu2” are created for "Zhang San" and "Li Si". Their student numbers are 201 and 202, and their grades are as follows: Mathematics 89 and 81, physics 75.5 and 77. Then, the function show() is called to display student name, student number, math score, physics score. Finally, the aver() is called to calculate and display the average score.c++编程
时间: 2024-02-05 09:02:24 浏览: 54
下面是C++代码实现。
```c++
#include <iostream>
#include <string>
using namespace std;
class CStudent {
private:
string stuName;
int stuNum;
double mathSco;
double phiSco;
public:
CStudent(string name, int num, double math, double phy);
~CStudent();
void myset(double math, double phy);
void show();
friend double aver(CStudent stu);
};
CStudent::CStudent(string name, int num, double math, double phy) {
stuName = name;
stuNum = num;
mathSco = math;
phiSco = phy;
}
CStudent::~CStudent() {}
void CStudent::myset(double math, double phy) {
mathSco = math;
phiSco = phy;
}
void CStudent::show() {
cout << "Student Name: " << stuName << endl;
cout << "Student Number: " << stuNum << endl;
cout << "Math Score: " << mathSco << endl;
cout << "Physics Score: " << phiSco << endl;
}
double aver(CStudent stu) {
return (stu.mathSco + stu.phiSco) / 2.0;
}
int main() {
CStudent stu1("Zhang San", 201, 89, 75.5);
CStudent stu2("Li Si", 202, 81, 77);
stu1.show();
cout << "Average Score: " << aver(stu1) << endl;
stu2.show();
cout << "Average Score: " << aver(stu2) << endl;
return 0;
}
```
程序输出结果如下:
```
Student Name: Zhang San
Student Number: 201
Math Score: 89
Physics Score: 75.5
Average Score: 82.25
Student Name: Li Si
Student Number: 202
Math Score: 81
Physics Score: 77
Average Score: 79
```
阅读全文
相关推荐
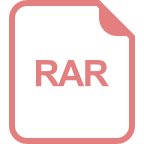
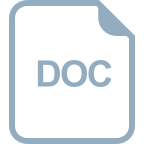















