能否提供一份简单的Java小游戏源代码示例,以便初学者理解?
时间: 2024-12-21 13:21:45 浏览: 7
当然可以。这里是一个简单的井字游戏(Tic Tac Toe)的Java源代码示例,它可以帮助初学者理解事件驱动编程和基本的游戏循环:
```java
import java.util.Scanner;
public class TicTacToe {
char[][] board = new char[3][3];
char currentPlayer = 'X';
void printBoard() {
for (int i = 0; i < 3; i++) {
for (char c : board[i]) {
System.out.print(c + " ");
}
System.out.println();
}
}
boolean placeMove(int row, int col) {
if (board[row][col] != '\0') return false;
board[row][col] = currentPlayer;
currentPlayer = (currentPlayer == 'X') ? 'O' : 'X';
return true;
}
public static void main(String[] args) {
TicTacToe game = new TicTacToe();
Scanner input = new Scanner(System.in);
while (!game.isGameOver()) {
game.printBoard();
System.out.println("Enter your move (row column):");
int row = input.nextInt() - 1;
int col = input.nextInt() - 1;
if (game.placeMove(row, col)) {
if (game.checkWin()) {
System.out.println("Player " + currentPlayer + " wins!");
break;
} else if (game.isBoardFull()) {
System.out.println("It's a tie!");
break;
}
}
}
input.close();
}
boolean isGameWon(char player) {
// Check rows, columns, and diagonals for a win
return ... // Implement the check conditions here
}
boolean isBoardFull() {
for (char[] row : board) {
for (char cell : row) {
if (cell == '\0') return false;
}
}
return true;
}
boolean isGameOver() {
return isGameWon('X') || isGameWon('O') || isBoardFull();
}
}
```
这个例子展示了创建一个棋盘、处理用户输入、检查游戏状态以及游戏结束条件的基本流程。你可以从这个简单的框架开始学习如何在Java中构建游戏。
阅读全文
相关推荐





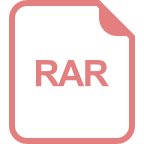







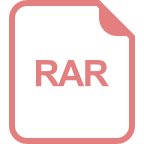




