编写一个C语言程序,以信号SIGTERM结束程序参数所指定的进程(通过进程号指定),并提示该进程是否被正确结束了。
时间: 2023-07-14 22:13:47 浏览: 135
以下是一个简单的示例代码,它接受一个进程号作为参数,并使用kill()函数发送SIGTERM信号来结束该进程。然后,使用waitpid()函数等待该进程结束,并输出相应的提示信息。
```c
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <signal.h>
#include <unistd.h>
#include <sys/wait.h>
int main(int argc, char *argv[])
{
if(argc != 2)
{
fprintf(stderr, "Usage: %s <pid>\n", argv[0]);
exit(EXIT_FAILURE);
}
pid_t pid = atoi(argv[1]);
if(kill(pid, SIGTERM) == -1)
{
fprintf(stderr, "Failed to send SIGTERM to process %d\n", pid);
exit(EXIT_FAILURE);
}
int status;
pid_t result = waitpid(pid, &status, 0);
if(result == -1)
{
fprintf(stderr, "Failed to wait for process %d\n", pid);
exit(EXIT_FAILURE);
}
if(WIFEXITED(status))
{
printf("Process %d exited normally with status %d\n", pid, WEXITSTATUS(status));
}
else if(WIFSIGNALED(status))
{
printf("Process %d terminated by signal %d\n", pid, WTERMSIG(status));
}
else
{
printf("Unknown status for process %d\n", pid);
}
return EXIT_SUCCESS;
}
```
请注意,这个程序需要使用root权限来结束其他进程。此外,如果指定的进程不存在或已经退出,程序可能会输出错误消息。
阅读全文
相关推荐


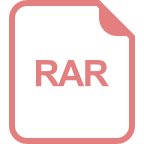
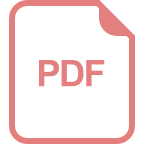
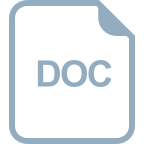
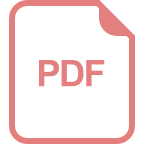
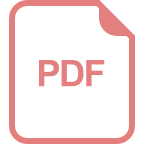
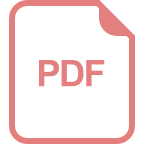
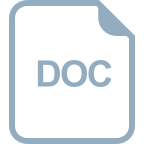
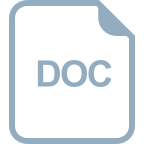
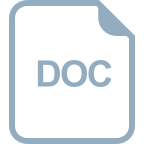
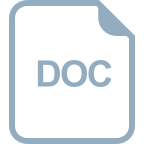
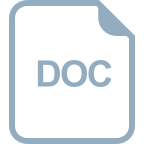


