编写一个程序,以信号SIGTERM结束程序参数所指定的进程(通过进程号指定),并提示该进程是否被正确结束了。c语言
时间: 2024-05-01 07:22:27 浏览: 53
以下是一个简单的C程序,可以通过进程号指定进程,并使用SIGTERM信号结束它。
```c
#include <stdio.h>
#include <signal.h>
#include <stdlib.h>
int main(int argc, char *argv[]) {
if (argc != 2) {
printf("Usage: %s <pid>\n", argv[0]);
exit(1);
}
int pid = atoi(argv[1]);
int ret = kill(pid, SIGTERM);
if (ret == 0) {
printf("Process %d terminated successfully\n", pid);
} else {
printf("Failed to terminate process %d\n", pid);
}
return 0;
}
```
使用方法:
```
$ gcc -o kill_process kill_process.c
$ ./kill_process <pid>
```
其中 `<pid>` 是要结束的进程的进程号。如果成功结束进程,则输出 `Process <pid> terminated successfully`,否则输出 `Failed to terminate process <pid>`。
相关问题
编写一个程序,以信号SIGTERM结束程序参数所指定的进程(通过进程号指定),并提示该进程是否被正确结束了。
以下是一个简单的Python程序,可以通过传入进程号,向指定进程发送 SIGTERM 信号,然后等待该进程结束,并输出该进程是否被正确结束的信息。
```python
import os
import signal
import sys
if len(sys.argv) < 2:
print("Usage: python terminate_process.py pid")
sys.exit(1)
pid = int(sys.argv[1])
try:
os.kill(pid, signal.SIGTERM)
print("Sent SIGTERM signal to process", pid)
except OSError as e:
print("Failed to send SIGTERM signal to process", pid, ":", e)
sys.exit(1)
try:
os.waitpid(pid, 0)
print("Process", pid, "successfully terminated")
except OSError as e:
print("Failed to terminate process", pid, ":", e)
sys.exit(1)
```
该程序首先通过 `sys.argv` 获取传入的参数,即需要结束的进程号。然后,使用 `os.kill()` 函数向指定进程发送 SIGTERM 信号。如果发送信号失败,则输出错误信息并退出程序。
如果成功发送信号,则使用 `os.waitpid()` 函数等待指定进程退出。如果进程正常退出,则输出成功信息;否则,输出错误信息。
你可以在命令行中运行该程序,例如:
```
python terminate_process.py 1234
```
其中,1234 是需要结束的进程号。
编写一个C语言程序,以信号SIGTERM结束程序参数所指定的进程(通过进程号指定),并提示该进程是否被正确结束了。
以下是一个简单的示例代码,它接受一个进程号作为参数,并使用kill()函数发送SIGTERM信号来结束该进程。然后,使用waitpid()函数等待该进程结束,并输出相应的提示信息。
```c
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <signal.h>
#include <unistd.h>
#include <sys/wait.h>
int main(int argc, char *argv[])
{
if(argc != 2)
{
fprintf(stderr, "Usage: %s <pid>\n", argv[0]);
exit(EXIT_FAILURE);
}
pid_t pid = atoi(argv[1]);
if(kill(pid, SIGTERM) == -1)
{
fprintf(stderr, "Failed to send SIGTERM to process %d\n", pid);
exit(EXIT_FAILURE);
}
int status;
pid_t result = waitpid(pid, &status, 0);
if(result == -1)
{
fprintf(stderr, "Failed to wait for process %d\n", pid);
exit(EXIT_FAILURE);
}
if(WIFEXITED(status))
{
printf("Process %d exited normally with status %d\n", pid, WEXITSTATUS(status));
}
else if(WIFSIGNALED(status))
{
printf("Process %d terminated by signal %d\n", pid, WTERMSIG(status));
}
else
{
printf("Unknown status for process %d\n", pid);
}
return EXIT_SUCCESS;
}
```
请注意,这个程序需要使用root权限来结束其他进程。此外,如果指定的进程不存在或已经退出,程序可能会输出错误消息。
阅读全文
相关推荐
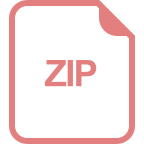
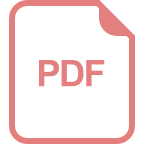
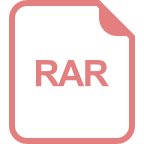













