doctrine/annotations 2.0 demo
时间: 2024-01-25 18:02:19 浏览: 133
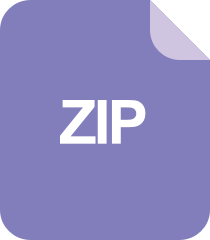
doctrine的demo
首先,你需要在你的项目中安装doctrine/annotations :
```
composer require doctrine/annotations
```
接下来,你需要创建一个注解类:
```php
namespace App\Annotation;
/**
* @Annotation
* @Target({"CLASS", "METHOD"})
*/
class MyAnnotation
{
public $value;
}
```
这是一个示例注解类,它被定义为可以用于类和方法上,并具有一个名为$value的属性。
接下来,你需要在你的代码中使用注解:
```php
use App\Annotation\MyAnnotation;
/**
* @MyAnnotation("Hello World")
*/
class MyClass
{
/**
* @MyAnnotation("My Method")
*/
public function myMethod()
{
// ...
}
}
```
这里我们使用了@MyAnnotation注解类,将其应用于MyClass类和myMethod()方法,并传递了一个字符串值"Hello World"和"My Method"。
最后,你可以使用Doctrine Annotations解析器来解析这些注解:
```php
use Doctrine\Common\Annotations\AnnotationReader;
$reader = new AnnotationReader();
$classAnnotations = $reader->getClassAnnotations(new ReflectionClass(MyClass::class));
foreach ($classAnnotations as $annotation) {
if ($annotation instanceof MyAnnotation) {
echo $annotation->value;
}
}
$methodAnnotations = $reader->getMethodAnnotations(new ReflectionMethod(MyClass::class, 'myMethod'));
foreach ($methodAnnotations as $annotation) {
if ($annotation instanceof MyAnnotation) {
echo $annotation->value;
}
}
```
在这里,我们使用Doctrine的AnnotationReader类来解析MyClass类和myMethod()方法中的注解。然后,我们循环遍历解析出的注解,并检查它们是否是我们的MyAnnotation类的实例。如果是,我们就可以访问它们的属性,例如$value。
阅读全文
相关推荐
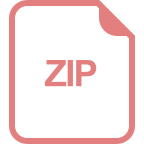




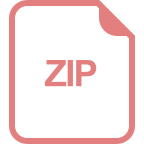
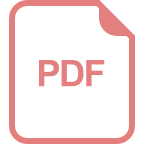
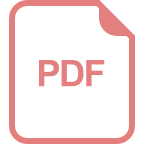
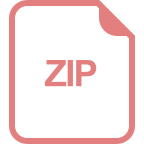
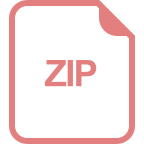
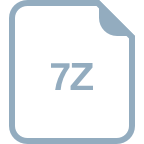
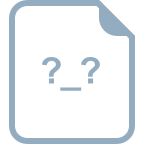
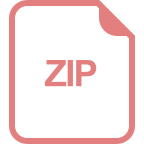
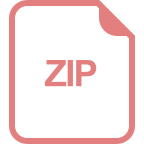
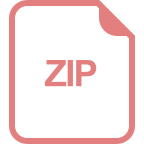
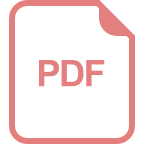
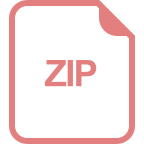