Linux 内核 spi驱动
时间: 2023-08-14 14:36:49 浏览: 70
Linux 内核提供了 SPI 总线驱动框架,用于支持 SPI 设备的驱动开发。SPI 总线驱动框架提供了一组 SPI 控制器驱动,用于管理硬件 SPI 控制器,并提供了一组 SPI 设备驱动,用于管理与 SPI 总线连接的设备。
在 Linux 内核中,SPI 总线驱动框架的核心文件是 `drivers/spi/spi.c`,它定义了 `spi_master` 结构体和一组操作函数,用于管理 SPI 总线和 SPI 设备。`spi_master` 结构体包含了指向 SPI 控制器驱动和 SPI 设备驱动的指针,以及一些与 SPI 总线相关的参数,如传输时钟频率、传输模式等。
SPI 控制器驱动需要实现一组操作函数,包括初始化、传输数据等。SPI 设备驱动需要实现一组操作函数,包括初始化、传输数据等。在初始化 SPI 设备驱动时,需要向 SPI 控制器驱动注册一个 `spi_device` 结构体,该结构体包含了 SPI 设备的相关信息,如设备名称、传输模式等。
以下是一个简单的 SPI 设备驱动的示例代码:
```
#include <linux/module.h>
#include <linux/spi/spi.h>
static int spi_example_probe(struct spi_device *spi)
{
// 初始化 SPI 设备
printk(KERN_INFO "spi_example_probe: %s\n", spi->modalias);
return 0;
}
static int spi_example_remove(struct spi_device *spi)
{
// 卸载 SPI 设备
printk(KERN_INFO "spi_example_remove: %s\n", spi->modalias);
return 0;
}
static struct spi_device_id spi_example_id[] = {
{"spi_example", 0},
{},
};
MODULE_DEVICE_TABLE(spi, spi_example_id);
static struct spi_driver spi_example_driver = {
.driver = {
.name = "spi_example",
.owner = THIS_MODULE,
},
.probe = spi_example_probe,
.remove = spi_example_remove,
.id_table = spi_example_id,
};
static int __init spi_example_init(void)
{
// 注册 SPI 设备驱动
int ret = spi_register_driver(&spi_example_driver);
if (ret < 0) {
printk(KERN_ERR "Failed to register SPI device driver\n");
}
return ret;
}
static void __exit spi_example_exit(void)
{
// 注销 SPI 设备驱动
spi_unregister_driver(&spi_example_driver);
}
module_init(spi_example_init);
module_exit(spi_example_exit);
MODULE_LICENSE("GPL");
MODULE_AUTHOR("Your Name");
MODULE_DESCRIPTION("SPI example driver");
```
在上面的代码中,`spi_example_probe` 和 `spi_example_remove` 函数分别用于初始化和卸载 SPI 设备。`spi_example_id` 定义了 SPI 设备驱动支持的设备 ID,`spi_example_driver` 定义了 SPI 设备驱动的相关信息,包括设备名称、初始化函数、卸载函数等。`spi_example_init` 和 `spi_example_exit` 函数分别用于注册和注销 SPI 设备驱动。
需要注意的是,上述代码仅为示例代码,实际开发中需要根据具体的硬件和应用场景进行修改和优化。
相关推荐
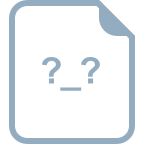
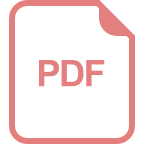
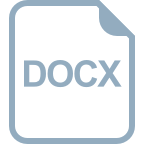














