写一个复杂的有地鼠图案,有背景图,而且地鼠随机出现的html打地鼠代码
时间: 2024-03-17 10:44:42 浏览: 13
好的,以下是一个稍微复杂一些的例子,实现了有背景图、地鼠随机出现的打地鼠游戏:
```html
<!DOCTYPE html>
<html>
<head>
<title>打地鼠游戏</title>
<style>
body {
background-image: url("background.jpg");
background-repeat: no-repeat;
background-size: cover;
margin: 0;
padding: 0;
}
.container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.mole {
background-image: url("mole.png");
background-size: contain;
width: 100px;
height: 100px;
opacity: 0;
transition: opacity 0.5s ease-in-out;
position: absolute;
top: 0;
left: 0;
right: 0;
bottom: 0;
margin: auto;
}
.show {
opacity: 1;
}
</style>
</head>
<body>
<div class="container"></div>
<script>
const container = document.querySelector(".container");
setInterval(() => {
// 随机生成 1~9 的整数,表示地鼠出现的位置
const position = Math.floor(Math.random() * 9) + 1;
// 创建地鼠元素
const mole = document.createElement("div");
mole.classList.add("mole");
// 将地鼠元素添加到容器中,并设置其出现位置
container.appendChild(mole);
switch (position) {
case 1:
mole.style.top = "10%";
mole.style.left = "10%";
break;
case 2:
mole.style.top = "10%";
mole.style.left = "50%";
break;
case 3:
mole.style.top = "10%";
mole.style.right = "10%";
break;
case 4:
mole.style.top = "50%";
mole.style.left = "10%";
break;
case 5:
mole.style.top = "50%";
mole.style.left = "50%";
break;
case 6:
mole.style.top = "50%";
mole.style.right = "10%";
break;
case 7:
mole.style.bottom = "10%";
mole.style.left = "10%";
break;
case 8:
mole.style.bottom = "10%";
mole.style.left = "50%";
break;
case 9:
mole.style.bottom = "10%";
mole.style.right = "10%";
break;
}
// 让地鼠出现一段时间后消失,并从容器中移除
setTimeout(() => {
mole.classList.remove("show");
setTimeout(() => {
container.removeChild(mole);
}, 500);
}, 1000);
}, 1500);
</script>
</body>
</html>
```
在这个例子中,我们使用了一个 `.container` 元素来容纳地鼠图案。我们定义了一个 `.mole` 类,用于表示地鼠图案。当地鼠出现时,我们会动态创建一个 `.mole` 元素,并将其添加到 `.container` 中。地鼠图案的出现位置是随机的,我们通过 switch 语句来设置其具体位置。
在 JavaScript 中,我们使用 `setInterval` 函数来定期生成地鼠。每个地鼠会在出现一段时间后消失,并从容器中移除。
相关推荐
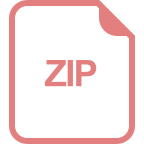
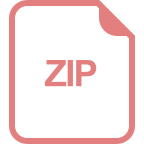
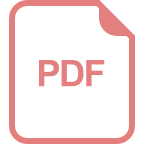













