C 语言实现通过脚本来定义数据结构体
时间: 2023-10-02 22:04:30 浏览: 134
在C语言中,我们可以使用预处理指令和宏来实现通过脚本来定义数据结构体。下面是一个简单的示例:
首先,我们可以创建一个文本文件(例如 "data_structure.txt"),在其中定义我们的数据结构。例如,我们定义一个名为 "Person" 的结构体,包含姓名和年龄两个字段。
```plaintext
data_structure.txt:
STRUCT_DEFINITION(Person)
FIELD_DEFINITION(char*, name)
FIELD_DEFINITION(int, age)
END_STRUCT_DEFINITION
```
接下来,我们可以创建一个C文件(例如 "data_structure_generator.c"),使用预处理指令和宏来解析并生成数据结构体的代码。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义结构体生成宏
#define STRUCT_DEFINITION(name) \
typedef struct { \
name##_FIELDS \
} name
// 定义字段生成宏
#define FIELD_DEFINITION(type, name) \
type name;
// 定义结束结构体生成宏
#define END_STRUCT_DEFINITION
// 解析文本文件生成数据结构体代码
void generateDataStructure() {
FILE* file = fopen("data_structure.txt", "r");
if (file == NULL) {
printf("Failed to open file.\n");
return;
}
char line[100];
char structName[100];
char structCode[1000];
strcpy(structCode, "");
while (fgets(line, sizeof(line), file)) {
if (strstr(line, "STRUCT_DEFINITION") != NULL) {
sscanf(line, "STRUCT_DEFINITION(%s)", structName);
} else if (strstr(line, "FIELD_DEFINITION") != NULL) {
char fieldType[100], fieldName[100];
sscanf(line, "FIELD_DEFINITION(%s, %s)", fieldType, fieldName);
strcat(structCode, fieldType);
strcat(structCode, " ");
strcat(structCode, fieldName);
strcat(structCode, ";\n");
} else if (strstr(line, "END_STRUCT_DEFINITION") != NULL) {
break;
}
}
fclose(file);
printf("Generated code:\n");
printf("--------------\n");
printf("typedef struct {\n");
printf("%s", structCode);
printf("} %s;\n", structName);
}
int main() {
generateDataStructure();
return 0;
}
```
运行 "data_structure_generator.c" 文件,它将解析 "data_structure.txt" 文件并生成相应的数据结构体代码。在这个例子中,它将生成一个名为 "Person" 的结构体,具有 "name" 和 "age" 两个字段。
```plaintext
Generated code:
--------------
typedef struct {
char* name;
int age;
} Person;
```
通过这种方式,我们可以通过脚本来定义数据结构体,并且可以根据需要在代码中生成相应的数据结构体。请注意,这只是一个简单的示例,实际应用中可能需要更复杂的解析和处理逻辑。
阅读全文
相关推荐
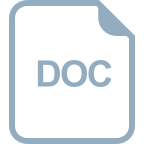
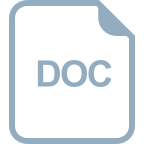
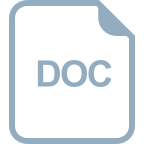
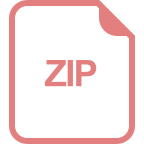
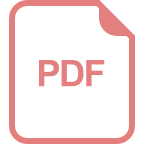
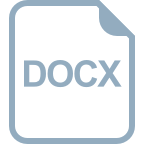
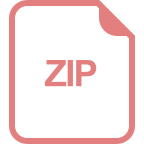
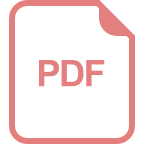
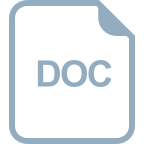
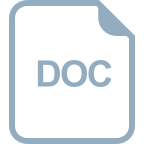
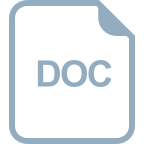
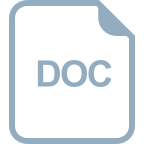
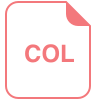
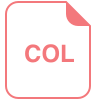
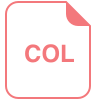
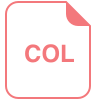
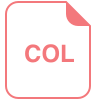
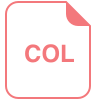
