在主函数中输入10个字符串。用另一函数对它们排序。然后在主函数输出这10个已排好序的字符串。要求用行指针实现。 函数接口定义: void sort(char (*p)[20]) ;
时间: 2023-09-12 21:07:29 浏览: 63
以下是实现代码:
```c
#include <stdio.h>
#include <string.h>
void sort(char (*p)[20]);
int main()
{
char str[10][20];
char (*p)[20] = str; // 行指针
printf("请输入10个字符串:\n");
for (int i = 0; i < 10; i++)
{
scanf("%s", *(p + i));
}
sort(p);
printf("排序后的字符串为:\n");
for (int i = 0; i < 10; i++)
{
printf("%s\n", *(p + i));
}
return 0;
}
void sort(char (*p)[20])
{
char temp[20];
for (int i = 0; i < 9; i++)
{
for (int j = i + 1; j < 10; j++)
{
if (strcmp(*(p + i), *(p + j)) > 0)
{
strcpy(temp, *(p + i));
strcpy(*(p + i), *(p + j));
strcpy(*(p + j), temp);
}
}
}
}
```
主函数中定义了一个二维字符数组 `str`,并通过行指针 `p` 指向该数组的首地址。然后通过循环输入10个字符串。
在 `sort` 函数中,采用选择排序的方式对字符串数组进行排序。排序完成后,再通过循环在主函数中输出已排好序的字符串。
阅读全文
相关推荐
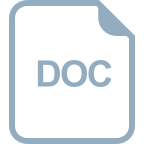
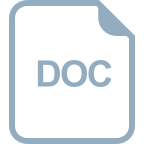
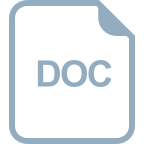














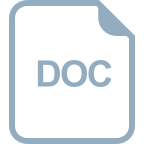
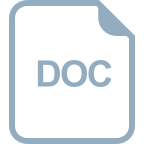