如何用Python需要写出屏保彩虹代码
时间: 2024-12-18 13:19:07 浏览: 8
编写一个简单的Python屏幕保护程序,比如显示彩虹动画效果,通常会涉及到使用pygame库来处理窗口和图形操作。以下是一个基础的示例,它会在屏幕上绘制出不断变化颜色的线条来模拟彩虹:
```python
import pygame
from random import randint
# 初始化pygame
pygame.init()
# 设置窗口大小
screen_width = 800
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
# 设置彩虹颜色列表
colors = [(255, 0, 0), (255, 255, 0), (0, 255, 0), (0, 255, 255), (0, 0, 255)]
# 游戏主循环
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT or (event.type == pygame.KEYDOWN and event.key == pygame.K_ESCAPE):
running = False
# 更新屏幕背景色
screen.fill((0, 0, 0))
# 绘制彩虹线条
for i in range(40): # 可调整线条数量
x1 = randint(0, screen_width)
y1 = randint(0, screen_height)
x2 = x1 + randint(1, screen_width // 2)
y2 = y1 + randint(1, screen_height // 2)
color_index = i % len(colors)
line_color = colors[color_index]
pygame.draw.line(screen, line_color, (x1, y1), (x2, y2), 2)
# 更新显示
pygame.display.flip()
pygame.time.delay(50) # 控制帧率,每50毫秒更新一次
# 关闭pygame
pygame.quit()
```
这个代码创建了一个基本的窗口,并在其中随机生成并移动颜色线段,形成了类似彩虹的效果。你可以根据需求修改颜色、线条样式以及动画速度。
阅读全文
相关推荐
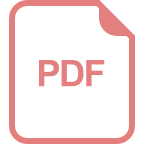
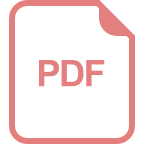
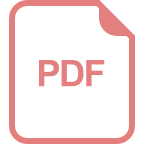















