isinstance(index, int) and 0
时间: 2024-09-20 12:10:26 浏览: 25
`isinstance(index, int)` 是 Python 中的一个内置函数,用于检查变量 `index` 是否是一个整数类型。如果 `index` 是整型,这个表达式的结果会是 `True`;如果不是,结果就是 `False`。
当你将 `and 0` 连接在一起时,这实际上是布尔运算符 "and" 的应用。在这个上下文中,`0` 表示一个常量值,其布尔值是 `False`(因为数字 0 被视为 False 在布尔运算中)。所以整个表达式的含义是,如果 `index` 是整数,它返回 `True`,否则,如果 `index` 不是整数,那么由于 `and` 操作符的短路特性(一旦前面条件为假,则不再计算后面的表达式),整个表达式结果为 `False` 并且相当于 `False and 0`,结果还是 `False`。
举个例子:
```python
>>> index = 5
>>> isinstance(index, int) and 0 # True
False
>>> index = 'hello'
>>> isinstance(index, int) and 0 # False because index is not an integer
False
```
相关问题
Implement fastest_words, which returns which words each player typed fastest. This function is called once both players have finished typing. It takes in a game. The game argument is a game data abstraction, like the one returned in Problem 9. You can access words in the game with selectors word_at, which takes in a game and the word_index (an integer). You can access the time it took any player to type any word using the time function provided in cats.py. The fastest_words function returns a list of lists of words, one list for each player, and within each list the words they typed the fastest. In the case of a tie, consider the earliest player in the list (the smallest player index) to be the one who typed it the fastest. Be sure to use the accessor functions for the game data abstraction, rather than assuming a particular data format.其中game函数如下def game(words, times): """A data abstraction containing all words typed and their times.""" assert all([type(w) == str for w in words]), 'words should be a list of strings' assert all([type(t) == list for t in times]), 'times should be a list of lists' assert all([isinstance(i, (int, float)) for t in times for i in t]), 'times lists should contain numbers' assert all([len(t) == len(words) for t in times]), 'There should be one word per time.' return
Sure, I can help you with that. Here's the implementation of the function fastest_words():
```
def fastest_words(game):
num_players = len(game.times)
num_words = len(game.words)
player_words = [[] for _ in range(num_players)]
for word_index in range(num_words):
fastest_player = 0
fastest_time = game.time(0, word_index)
for player_index in range(1, num_players):
time = game.time(player_index, word_index)
if time < fastest_time:
fastest_player = player_index
fastest_time = time
player_words[fastest_player].append(game.word_at(word_index))
return player_words
```
This function takes in a game data abstraction and returns a list of lists of words, one list for each player, and within each list the words they typed the fastest. The function first initializes an empty list for each player to store their fastest words. It then loops through each word in the game and finds the player who typed the word the fastest. If there's a tie, the function considers the earliest player in the list to be the one who typed it the fastest.
I hope this helps! Let me know if you have any other questions.
输出结果报错:TypeError: '<' not supported between instances of 'int' and 'list' 是为什么
这个错误 "TypeError: '<' not supported between instances of 'int' and 'list'" 表示你试图在一个整数和一个列表之间使用小于 (`<`) 操作符进行比较。Python 不支持不同类型的对象之间的比较,特别是当一个是基本类型(如整数、浮点数等),另一个是复合数据类型(如列表、字典等)时。
例如,在你的代码中,可能有这样的情况:
```python
current_value = 10
sorted_list = [1, 2, 3]
if current_value < sorted_list: # 错误操作
```
在这个例子中,`current_value` 是一个整数,而 `sorted_list` 是一个列表。尝试将整数 `10` 和列表 `[1, 2, 3]` 相比显然是无效的,所以会抛出 `TypeError`。
为了修复这个问题,你需要确保你只在相同类型的对象之间执行比较。如果 `current_value` 应该与列表中的某个元素进行比较,那么你应该明确地访问列表的相应位置,而不是直接将整个列表当作整体进行比较。例如:
```python
if current_value < sorted_list: # 如果你想比较第一个元素
```
或者,如果 `current_value` 是一个搜索条件,你可以先将其转换为列表的一个元素,然后再做比较:
```python
if isinstance(current_value, int):
index = sorted_list.index(current_value) if current_value in sorted_list else None
...
```
总之,确保你的比较操作是在同类型的数据结构上执行的。
阅读全文
相关推荐












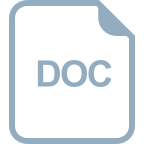


