c++顺序高斯消元法并计算
时间: 2024-10-23 21:18:18 浏览: 25
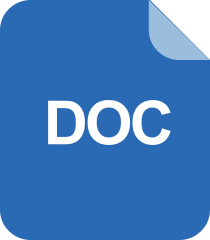
VC编程实现高斯先列主元消元法求解线性方程组
在C++中,顺序高斯消元法通常用于求解线性方程组。它涉及一系列矩阵操作,包括行交换和行缩放,直到矩阵变为上三角形式。下面是一个简单的例子,展示如何用C++编写顺序高斯消元算法来计算一组线性方程组的解:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
// 行交换函数
void swap_rows(int& i, int& j, std::vector<std::vector<int>>& A) {
std::swap(A[i], A[j]);
}
// 行缩放函数
void scale_row(int i, double scalar, std::vector<std::vector<int>>& A) {
for (size_t j = i; j < A[i].size(); ++j) {
A[i][j] *= scalar;
}
}
// 消元过程,假设A是m x n矩阵,b是m维列向量
std::vector<double> solve_gauss_elimination(const std::vector<std::vector<int>>& A, const std::vector<int>& b) {
int m = A.size(), n = A[0].size();
// 确保方程组有解(即系数矩阵的秩等于未知数的数量)
if (m != n) {
throw std::runtime_error("Matrix is not square, cannot solve.");
}
// 创建身份矩阵I作为初始猜测解
std::vector<double> solution(m, 0.0);
for (int i = 0; i < m; ++i) {
solution[i] = b[i];
}
// 执行高斯消元
for (int i = 0; i < m - 1; ++i) {
// 找到第一个非零元素所在的列
int pivot_col = i;
for (int j = i + 1; j < n; ++j) {
if (A[i][j] != 0) {
pivot_col = j;
break;
}
}
// 将当前行与含有非零元素的行交换
if (pivot_col > i) {
swap_rows(i, pivot_col, A);
std::swap(solution[i], solution[pivot_col]);
}
// 除以第一列的非零元素
scale_row(i, 1.0 / A[i][i], A);
scale_row(i, 1.0 / A[i][i], solution);
// 对其余行进行消元
for (int j = i + 1; j < m; ++j) {
scale_row(j, -A[j][i], A);
scale_row(j, -A[j][i], solution);
}
}
return solution;
}
int main() {
std::vector<std::vector<int>> A = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
std::vector<int> b = {10, 20, 30};
try {
auto solution = solve_gauss_elimination(A, b);
for (double s : solution) {
std::cout << "x_" << s << " = " << s << std::endl;
}
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
```
在这个例子中,`solve_gauss_elimination`函数接收一个系数矩阵`A`和常数项向量`b`,然后返回解向量`solution`。如果方程组无解或者系数矩阵奇异(即行列式为0),函数会抛出异常。
阅读全文
相关推荐
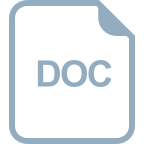
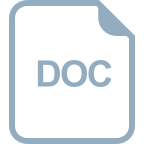
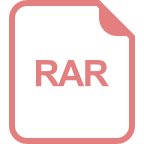
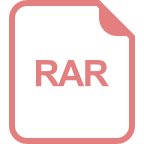

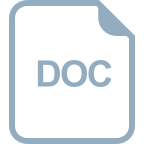
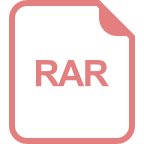
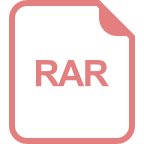
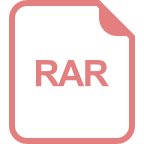
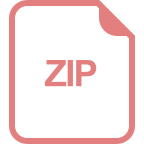
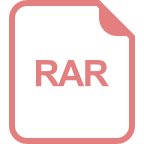
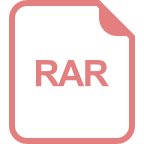
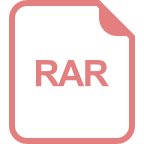
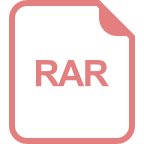
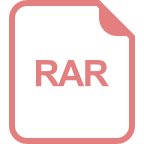
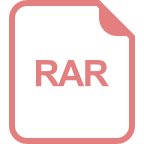

