pyspark使用withColumn如何指定字段的类型
时间: 2024-04-29 15:21:24 浏览: 17
使用withColumn时,可以使用cast函数来指定字段的类型。例如,如果要将一个名为"age"的字段转换为integer类型,可以使用下面的语句:
```
from pyspark.sql.functions import col
df = df.withColumn("age", col("age").cast("integer"))
```
其中,"age"为目标字段名,col("age")表示选择原始字段,cast("integer")指定了要转换的类型。
相关问题
使用withColumn加一个数组字段
可以使用Spark DataFrame的`withColumn`函数结合`array`函数来添加一个数组字段。下面是一个示例代码:
```python
from pyspark.sql.functions import array, lit
# 创建一个DataFrame示例
df = spark.createDataFrame([(1, "a"), (2, "b"), (3, "c")], ["id", "name"])
# 使用withColumn添加一个数组字段
new_df = df.withColumn("new_col", array(lit("x"), lit("y"), lit("z")))
# 显示结果
new_df.show()
```
在上面的示例代码中,我们首先创建了一个包含两个列(`id`和`name`)的DataFrame。然后,我们使用`withColumn`函数添加了一个名为`new_col`的新列,该列包含一个包含三个字符串(`x`、`y`和`z`)的数组。最后,我们使用`show`函数显示了新的DataFrame。
pyspark explode函数的使用
pyspark中的explode函数用于将包含数组或者Map类型的字段拆分成多行。它会将数组或Map中的每个元素拆分成一行,并复制其他列的值。
使用explode函数的基本语法如下:
```python
from pyspark.sql.functions import explode
df.withColumn('new_column', explode('array_column'))
```
其中,`df`是你的DataFrame对象,`array_column`是包含数组或Map的列名,`new_column`是你希望生成的新列的名称。
示例:
假设有一个DataFrame `df`,包含两列:`id`和`colors`。`colors`列是一个包含多个颜色的数组。你可以使用explode函数将每个颜色拆分成单独的行,如下所示:
```python
from pyspark.sql import SparkSession
from pyspark.sql.functions import explode
# 创建SparkSession
spark = SparkSession.builder.getOrCreate()
# 创建示例数据
data = [(1, ["red", "green", "blue"]), (2, ["yellow", "orange"])]
# 创建DataFrame
df = spark.createDataFrame(data, ["id", "colors"])
# 使用explode函数拆分数组
df_exploded = df.withColumn("color", explode("colors"))
df_exploded.show()
```
输出结果如下:
```
+---+------------------+-----+
| id| colors|color|
+---+------------------+-----+
| 1|[red, green, blue]| red|
| 1|[red, green, blue]|green|
| 1|[red, green, blue]| blue|
| 2| [yellow, orange]|yellow|
| 2| [yellow, orange]|orange|
+---+------------------+-----+
```
可以看到,`colors`列中的每个颜色都被拆分成了单独的行,并生成了新的`color`列。
相关推荐
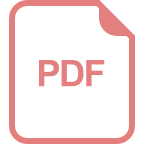
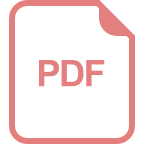













