java AES和RSA加密UTIL
时间: 2023-08-26 21:15:30 浏览: 168
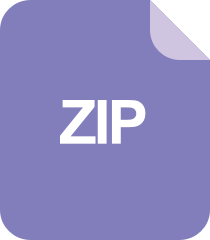
AES+RSA加密解密(js和java互通)

以下是 Java 中使用 AES 和 RSA 加密的示例代码:
AES 加密:
```java
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import java.util.Base64;
public class AESUtil {
private static final String ALGORITHM = "AES";
private static final String CIPHER_ALGORITHM = "AES/ECB/PKCS5Padding";
private static final byte[] KEY = "your-secret-key".getBytes();
public static String encrypt(String input) throws Exception {
SecretKeySpec secretKeySpec = new SecretKeySpec(KEY, ALGORITHM);
Cipher cipher = Cipher.getInstance(CIPHER_ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, secretKeySpec);
byte[] encrypted = cipher.doFinal(input.getBytes());
return Base64.getEncoder().encodeToString(encrypted);
}
}
```
RSA 加密:
```java
import java.security.KeyFactory;
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.PrivateKey;
import java.security.PublicKey;
import java.security.spec.PKCS8EncodedKeySpec;
import java.security.spec.X509EncodedKeySpec;
import javax.crypto.Cipher;
import java.util.Base64;
public class RSAUtil {
private static final String ALGORITHM = "RSA";
private static final String CIPHER_ALGORITHM = "RSA/ECB/PKCS1Padding";
private static final int KEY_SIZE = 2048;
public static KeyPair generateKeyPair() throws Exception {
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance(ALGORITHM);
keyPairGenerator.initialize(KEY_SIZE);
return keyPairGenerator.generateKeyPair();
}
public static String encrypt(String input, PublicKey publicKey) throws Exception {
Cipher cipher = Cipher.getInstance(CIPHER_ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
byte[] encrypted = cipher.doFinal(input.getBytes());
return Base64.getEncoder().encodeToString(encrypted);
}
public static PrivateKey decodePrivateKey(String key) throws Exception {
byte[] keyBytes = Base64.getDecoder().decode(key.getBytes());
PKCS8EncodedKeySpec keySpec = new PKCS8EncodedKeySpec(keyBytes);
KeyFactory keyFactory = KeyFactory.getInstance(ALGORITHM);
return keyFactory.generatePrivate(keySpec);
}
public static PublicKey decodePublicKey(String key) throws Exception {
byte[] keyBytes = Base64.getDecoder().decode(key.getBytes());
X509EncodedKeySpec keySpec = new X509EncodedKeySpec(keyBytes);
KeyFactory keyFactory = KeyFactory.getInstance(ALGORITHM);
return keyFactory.generatePublic(keySpec);
}
}
```
注意:这些代码只是示例,并不是完整且安全的加密实现。在实际使用中,需要根据具体的需求和安全要求进行修改和优化。
阅读全文
相关推荐
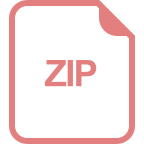
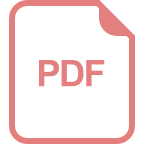
















