用c语言实现单链表的ADT,其中要包括链表的生成、销毁、插入、删除、查找
时间: 2023-08-09 21:11:44 浏览: 40
好的,以下是用C语言实现单链表ADT的示例代码:
```c
// 定义链表节点结构体
typedef struct ListNode {
int data; // 节点数据
struct ListNode *next; // 指向下一个节点的指针
} ListNode;
// 创建一个新节点
ListNode* createNode(int data) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// 销毁链表
void destroyList(ListNode* head) {
ListNode* curNode = head;
while (curNode != NULL) {
ListNode* nextNode = curNode->next;
free(curNode);
curNode = nextNode;
}
}
// 在链表尾部插入一个新节点
void insertNode(ListNode* head, int data) {
ListNode* newNode = createNode(data);
ListNode* curNode = head;
while (curNode->next != NULL) {
curNode = curNode->next;
}
curNode->next = newNode;
}
// 删除链表中第一个值为data的节点
void deleteNode(ListNode* head, int data) {
ListNode* curNode = head;
ListNode* prevNode = NULL;
while (curNode != NULL && curNode->data != data) {
prevNode = curNode;
curNode = curNode->next;
}
if (curNode != NULL) {
if (prevNode != NULL) {
prevNode->next = curNode->next;
} else {
head = curNode->next;
}
free(curNode);
}
}
// 查找链表中第一个值为data的节点
ListNode* findNode(ListNode* head, int data) {
ListNode* curNode = head;
while (curNode != NULL && curNode->data != data) {
curNode = curNode->next;
}
return curNode;
}
```
在使用时,可以先创建一个头节点,然后调用上述函数进行链表的生成、销毁、插入、删除、查找等操作。例如:
```c
int main() {
// 创建头节点
ListNode* head = createNode(0);
// 在链表尾部插入节点
insertNode(head, 1);
insertNode(head, 2);
insertNode(head, 3);
// 查找节点
ListNode* node = findNode(head, 2);
if (node != NULL) {
printf("Found node: %d\n", node->data);
} else {
printf("Node not found.\n");
}
// 删除节点
deleteNode(head, 2);
// 销毁链表
destroyList(head);
return 0;
}
```
相关推荐
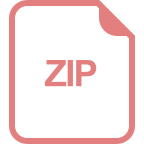
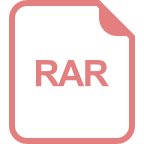














