点击复制后,将打开C知道体验页 | 用c语言实现单链表的ADT,其中要包括链表的生成、销毁、插入、删除、查找,并且注意代码的缩进
时间: 2023-05-12 08:03:05 浏览: 78
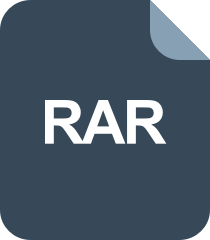
数据结构单链表的ADT用c实现。。
以下是用C语言实现单链表的ADT的代码,包括链表的生成、销毁、插入、删除和查找。请注意代码的缩进。
```
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node *next;
} Node;
typedef struct List {
Node *head;
int size;
} List;
List *createList() {
List *list = (List *)malloc(sizeof(List));
list->head = NULL;
list->size = 0;
return list;
}
void destroyList(List *list) {
Node *current = list->head;
while (current != NULL) {
Node *next = current->next;
free(current);
current = next;
}
free(list);
}
void insert(List *list, int data) {
Node *newNode = (Node *)malloc(sizeof(Node));
newNode->data = data;
newNode->next = list->head;
list->head = newNode;
list->size++;
}
void delete(List *list, int data) {
Node *current = list->head;
Node *previous = NULL;
while (current != NULL) {
if (current->data == data) {
if (previous == NULL) {
list->head = current->next;
} else {
previous->next = current->next;
}
free(current);
list->size--;
return;
}
previous = current;
current = current->next;
}
}
Node *find(List *list, int data) {
Node *current = list->head;
while (current != NULL) {
if (current->data == data) {
return current;
}
current = current->next;
}
return NULL;
}
int main() {
List *list = createList();
insert(list, 1);
insert(list, 2);
insert(list, 3);
Node *node = find(list, 2);
if (node != NULL) {
printf("Found node with data: %d\n", node->data);
} else {
printf("Node not found\n");
}
delete(list, 2);
node = find(list, 2);
if (node != NULL) {
printf("Found node with data: %d\n", node->data);
} else {
printf("Node not found\n");
}
destroyList(list);
return 0;
}
```
阅读全文
相关推荐
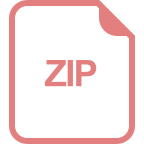
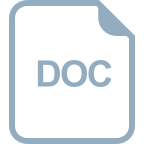
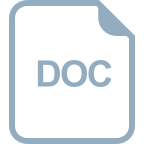
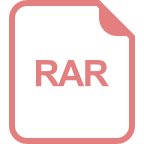
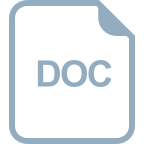
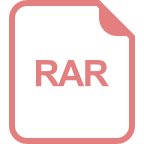
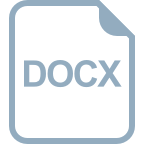
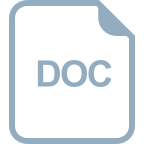
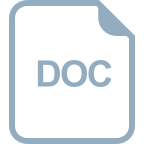
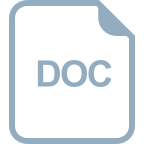
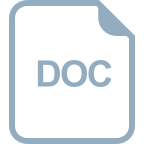
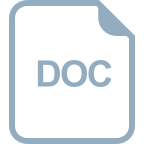
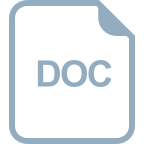
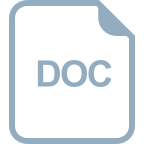
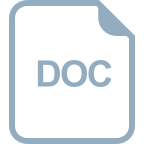
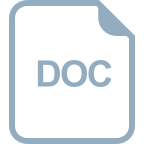
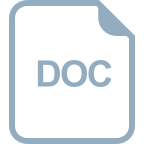