C语言如何利用pthreads库创建线程?
时间: 2024-09-25 07:07:37 浏览: 24
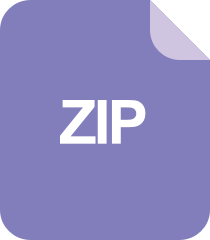
基于C语言实现的(控制台)用户级线程库【100010648】
在C语言中,使用pthreads(POSIX Threads)库创建线程通常需要以下几个步骤:
1. **包含头文件**:首先在源文件中包含`pthread.h`头文件,它包含了所有与线程相关的函数声明。
```c
#include <pthread.h>
```
2. **定义线程函数**:这是每个线程将执行的任务,通常接收一个指向数据结构的指针作为参数,并通过`void *thread_func(void *arg)`这样的形式定义。
3. **创建线程**:使用`pthread_create()`函数创建新线程。这个函数需要四个参数:
- `pthread_t *thread`:返回线程标识符的指针。
- `pthread_attr_t *attr`:线程属性设置,可以指定优先级、栈大小等,这里一般设为NULL表示默认属性。
- `void *(*start_routine)(void *)`:线程函数的地址。
- `void *arg`:传给线程函数的参数。
示例:
```c
pthread_t thread_id;
pthread_attr_t attr;
pthread_create(&thread_id, &attr, thread_func, (void*)data);
```
4. **同步线程**:如果需要控制线程之间的交互,可以使用互斥锁(mutex)、条件变量(condition variable)等同步工具,如`pthread_mutex_lock()`和`pthread_cond_wait()`。
5. **销毁线程**:完成任务后,需要调用`pthread_join()`等待线程结束,然后清理资源。
```c
pthread_join(thread_id, NULL); // 等待线程结束
pthread_exit(NULL); // 如果线程自身结束,释放资源
```
6. **处理错误**:务必检查`pthread_create()`和`pthread_join()`等函数的返回值,因为它们可能会返回错误码。
```c
if (pthread_create(&thread_id, &attr, thread_func, (void*)data) != 0) {
// 错误处理
}
```
阅读全文
相关推荐
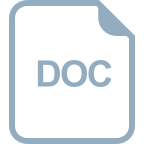
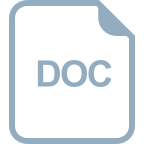
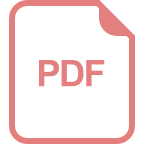

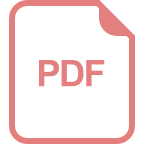
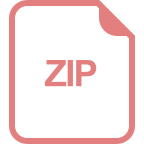
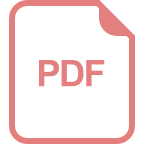
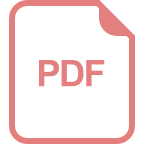
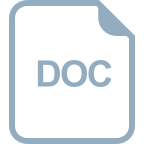
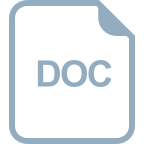
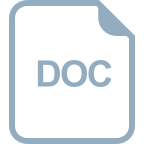






