新浪财经新闻爬取并进行舆情分析的代码
时间: 2023-06-23 22:09:15 浏览: 167
以下是一个简单的例子,使用Python和BeautifulSoup爬取新浪财经新闻并进行情感分析。
首先,需要安装必要的库:BeautifulSoup、requests和TextBlob(进行情感分析)。
```python
from textblob import TextBlob
import requests
from bs4 import BeautifulSoup
```
接下来,定义一个函数来获取新浪财经网站上的新闻标题和内容:
```python
def get_news(url):
# 发送请求
response = requests.get(url)
# 解析HTML
soup = BeautifulSoup(response.text, 'html.parser')
# 获取新闻标题
title = soup.find('h1', class_='main-title').text
# 获取新闻内容
content = ''
for p in soup.find_all('p'):
content += p.text
return title, content
```
接下来,定义一个函数来进行情感分析:
```python
def analyze_sentiment(text):
# 使用TextBlob进行情感分析
blob = TextBlob(text)
sentiment_score = blob.sentiment.polarity
if sentiment_score > 0:
return 'positive'
elif sentiment_score < 0:
return 'negative'
else:
return 'neutral'
```
最后,将所有代码放在一起:
```python
from textblob import TextBlob
import requests
from bs4 import BeautifulSoup
def get_news(url):
# 发送请求
response = requests.get(url)
# 解析HTML
soup = BeautifulSoup(response.text, 'html.parser')
# 获取新闻标题
title = soup.find('h1', class_='main-title').text
# 获取新闻内容
content = ''
for p in soup.find_all('p'):
content += p.text
return title, content
def analyze_sentiment(text):
# 使用TextBlob进行情感分析
blob = TextBlob(text)
sentiment_score = blob.sentiment.polarity
if sentiment_score > 0:
return 'positive'
elif sentiment_score < 0:
return 'negative'
else:
return 'neutral'
# 测试
url = 'https://finance.sina.com.cn/stock/usstock/c/2022-05-11/doc-imcwipii6960623.shtml'
title, content = get_news(url)
sentiment = analyze_sentiment(content)
print('标题:', title)
print('情感分析:', sentiment)
```
这个例子只是一个简单的示例,还有很多可以改进的地方。例如,可以使用更高级的情感分析算法,或者使用多线程/多进程来加快爬取速度等等。
阅读全文
相关推荐
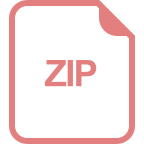
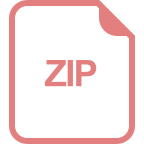
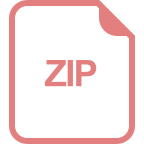
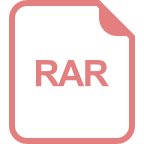
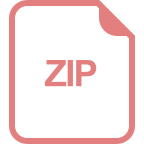
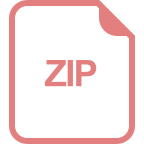
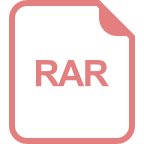
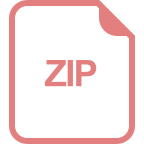
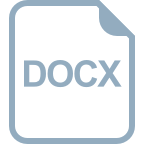
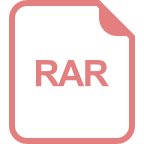
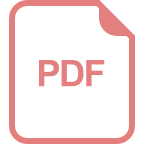
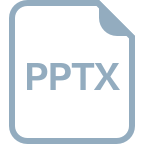
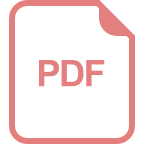