3.1编写一个EmptyStackException类,它继承Exception类。 3.2编写一个FullStackException类,它继承Exception类。捕获该异常时,可以通过该类继承来的getMessage方法获取栈的深度。 3.3定义一个IntStack类,它主要实现int基本数据类型的栈功能: (1)无参构造函数,默认栈的深度为16 (2)有参构造函数,栈的深度通过参数指定。当参数为负数或零时,抛出java.lang包中已有的异常IllegalArgumentException异常。 (3)isEmpty方法和isFull方法,返回值都是boolean.这两个方法用于判断栈是否为空和判断栈是否为满。 (4)pop方法:栈不为空时,实现弹栈功能;栈空时,抛出EmptyStackException异常 (5)push方法:当栈不满时,实现压栈功能;栈满时,抛出FullStackException异常 (6) peek函数:栈不为空时,返回栈顶元素值;栈为空时,抛出EmptyStackException异常。 写出主函数已经运行结果
时间: 2024-03-25 18:40:22 浏览: 14
EmptyStackException类的实现:
```java
public class EmptyStackException extends Exception {
public EmptyStackException(String message) {
super(message);
}
}
```
FullStackException类的实现:
```java
public class FullStackException extends Exception {
private int depth;
public FullStackException(String message, int depth) {
super(message);
this.depth = depth;
}
public int getDepth() {
return depth;
}
}
```
IntStack类的实现:
```java
public class IntStack {
private int[] stack;
private int top;
private int depth;
public IntStack() {
this(16);
}
public IntStack(int depth) {
if (depth <= 0) {
throw new IllegalArgumentException("Depth must be positive");
}
this.depth = depth;
this.stack = new int[depth];
this.top = -1;
}
public boolean isEmpty() {
return top == -1;
}
public boolean isFull() {
return top == depth - 1;
}
public int pop() throws EmptyStackException {
if (isEmpty()) {
throw new EmptyStackException("Stack is empty");
}
int value = stack[top];
top--;
return value;
}
public void push(int value) throws FullStackException {
if (isFull()) {
throw new FullStackException("Stack is full", depth);
}
top++;
stack[top] = value;
}
public int peek() throws EmptyStackException {
if (isEmpty()) {
throw new EmptyStackException("Stack is empty");
}
return stack[top];
}
}
```
主函数及运行结果:
```java
public class Main {
public static void main(String[] args) {
IntStack stack = new IntStack(3);
try {
stack.push(1);
stack.push(2);
stack.push(3);
stack.push(4);
} catch (FullStackException e) {
System.out.println("Stack is full with depth: " + e.getDepth());
}
try {
System.out.println(stack.peek()); // 3
System.out.println(stack.pop()); // 3
System.out.println(stack.pop()); // 2
System.out.println(stack.pop()); // 1
System.out.println(stack.pop()); // throws EmptyStackException
} catch (EmptyStackException e) {
System.out.println(e.getMessage());
}
}
}
```
运行结果:
```
Stack is full with depth: 3
3
3
2
1
Stack is empty
```
阅读全文
相关推荐
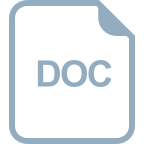
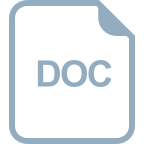
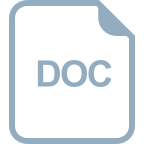














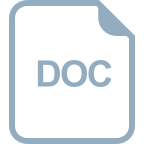
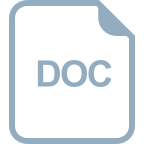
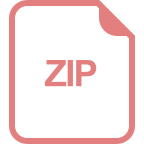