顺序表基本操作——学生成绩信息管理 【问题描述】 定义一个包含学生成绩(学号,姓名,成绩)的顺序表,读入相应的成绩信息来完成学生成绩表的创建。然后,(1)查找统计不及格的学生人数,(2)删除并以学号升序方式逐行输出不及格的学生信息。 【输入形式】 输入n+1行,其中第1行是学生人数,后n行是n本图书的信息(学号,姓名,成绩),每条信息占一行,学号、姓名、成绩使用空格分隔,成绩之后没有空格。 【输出形式】 输出数据有m+1行,其中第1行是不及格学生人数,后m行是不及格学生信息,每条信息占一行,学号、姓名、成绩使用空格分隔,成绩之后没有空格。 【样例输入】 3 1001 zhangsan 34 1002 wangwu 66 1003 lisi 56 【样例输出】 2 1001 zhangsan 34 1003 lisi 56 【样例说明】 如果不存在不及格同学,则输出提示信息“not found” 编写C++代码
时间: 2023-11-27 14:05:26 浏览: 133
好的,下面是解决这个问题的 C++ 代码,注释已经详细说明了每一步的操作:
```cpp
#include<iostream>
#include<string>
#include<algorithm>
using namespace std;
// 学生信息结构体
struct Student {
int id; // 学号
string name; // 姓名
int score; // 成绩
};
// 判断是否及格的函数
bool isPass(Student stu) {
return (stu.score >= 60);
}
// 比较函数,用于排序
bool cmp(Student stu1, Student stu2) {
return (stu1.id < stu2.id);
}
int main() {
int n;
cin >> n;
// 创建学生信息顺序表
Student* students = new Student[n];
for (int i = 0; i < n; i++) {
cin >> students[i].id >> students[i].name >> students[i].score;
}
// 统计不及格学生人数
int cnt = count_if(students, students + n, [](Student stu) {return !isPass(stu); });
if (cnt == 0) {
cout << "not found" << endl;
return 0;
}
// 删除不及格学生信息并按照学号升序输出
sort(students, students + n, cmp);
cout << cnt << endl;
for (int i = 0; i < n; i++) {
if (!isPass(students[i])) {
cout << students[i].id << " " << students[i].name << " " << students[i].score << endl;
}
}
return 0;
}
```
希望对你有帮助!
阅读全文
相关推荐


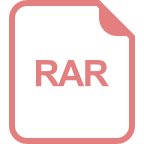


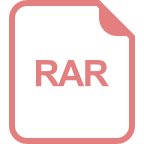



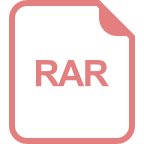
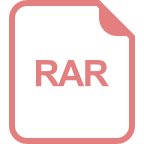
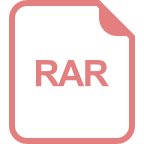




