用C语言写:采用树表示一个家谱关系,采用链表(孩子表示法)作为存储结构,实现家谱关系的相关运算。每个节点信息包含有:姓名、性别、配偶姓名、身份证号、年龄、出生日期等。 功能要求: 1)输入家族信息(要求自己设计文件存储格式,存放当前所有家族信息)2)输出家族信息(要求界面输出格式直观明了) 3)查找某人的所有儿子 4)查找某人的双亲 输出形式要有合理的提示,每个功能可以设立菜单,根据提示,可以完成相关的功能要求。 存储结构: 学生自己根据系统功能要求自己设计,但是要求家谱系统的相关数据要存储在数据文件中。
时间: 2024-02-19 16:00:20 浏览: 32
好的,以下是一个简单的实现。在这个实现中,我们使用了链表(孩子表示法)作为存储结构,同时利用了文件来存储家族信息。请注意,这个实现可能有一些限制,比如每个人只能有一个配偶,每个人的身份证号码必须唯一等等,您可以根据自己的需求进行修改。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NAME_LEN 20
#define MAX_ID_LEN 20
typedef struct _Node {
char name[MAX_NAME_LEN];
char sex;
char spouse[MAX_NAME_LEN];
char id[MAX_ID_LEN];
int age;
char birth[20];
struct _Node *child;
struct _Node *sibling;
} Node;
void save(Node *root, FILE *fp) {
if (root == NULL) {
return;
}
fwrite(root, sizeof(Node), 1, fp);
save(root->child, fp);
save(root->sibling, fp);
}
Node *load(FILE *fp) {
Node *root = NULL;
Node *prev = NULL;
Node *curr = NULL;
while (!feof(fp)) {
curr = (Node *) malloc(sizeof(Node));
if (fread(curr, sizeof(Node), 1, fp) != 1) {
free(curr);
break;
}
if (root == NULL) {
root = curr;
} else if (prev->child == NULL) {
prev->child = curr;
} else {
prev->sibling = curr;
}
prev = curr;
curr->child = NULL;
curr->sibling = NULL;
}
return root;
}
void print_node(Node *node) {
printf("Name: %s\n", node->name);
printf("Sex: %c\n", node->sex);
printf("Spouse: %s\n", node->spouse);
printf("ID: %s\n", node->id);
printf("Age: %d\n", node->age);
printf("Birth: %s\n", node->birth);
}
void print_tree(Node *root, int level) {
if (root == NULL) {
return;
}
for (int i = 0; i < level; i++) {
printf(" ");
}
print_node(root);
print_tree(root->child, level + 1);
print_tree(root->sibling, level);
}
Node *find_node(Node *root, char *name) {
if (root == NULL) {
return NULL;
}
if (strcmp(root->name, name) == 0) {
return root;
}
Node *child = find_node(root->child, name);
if (child != NULL) {
return child;
}
return find_node(root->sibling, name);
}
void find_sons(Node *node) {
if (node == NULL) {
printf("Not found.\n");
return;
}
if (node->child == NULL) {
printf("No sons.\n");
return;
}
printf("Sons: ");
Node *p = node->child;
while (p != NULL) {
printf("%s ", p->name);
p = p->sibling;
}
printf("\n");
}
void find_parents(Node *root, Node *node) {
if (root == NULL || node == NULL) {
printf("Not found.\n");
return;
}
if (root->child == node) {
printf("Father: %s\n", root->name);
printf("Mother: %s\n", root->spouse);
return;
}
find_parents(root->child, node);
find_parents(root->sibling, node);
}
int main() {
FILE *fp = fopen("family.dat", "rb");
Node *root = NULL;
if (fp != NULL) {
root = load(fp);
fclose(fp);
}
int choice = -1;
char name[MAX_NAME_LEN];
Node *node = NULL;
while (choice != 0) {
printf("1. Add family member\n");
printf("2. Print family tree\n");
printf("3. Find sons\n");
printf("4. Find parents\n");
printf("0. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
node = (Node *) malloc(sizeof(Node));
printf("Name: ");
scanf("%s", node->name);
printf("Sex (M/F): ");
scanf(" %c", &node->sex);
printf("Spouse: ");
scanf("%s", node->spouse);
printf("ID: ");
scanf("%s", node->id);
printf("Age: ");
scanf("%d", &node->age);
printf("Birth (YYYY-MM-DD): ");
scanf("%s", node->birth);
node->child = NULL;
node->sibling = NULL;
if (root == NULL) {
root = node;
} else {
printf("Parent's name: ");
scanf("%s", name);
Node *parent = find_node(root, name);
if (parent == NULL) {
printf("Parent not found.\n");
free(node);
break;
}
if (parent->child == NULL) {
parent->child = node;
} else {
Node *p = parent->child;
while (p->sibling != NULL) {
p = p->sibling;
}
p->sibling = node;
}
}
fp = fopen("family.dat", "wb");
save(root, fp);
fclose(fp);
break;
case 2:
print_tree(root, 0);
break;
case 3:
printf("Name: ");
scanf("%s", name);
node = find_node(root, name);
find_sons(node);
break;
case 4:
printf("Name: ");
scanf("%s", name);
node = find_node(root, name);
find_parents(root, node);
break;
case 0:
break;
default:
printf("Invalid choice.\n");
break;
}
}
return 0;
}
```
在这个实现中,我们定义了一个名为`Node`的结构体,其中包含了家族成员的各项信息,以及指向其子节点和兄弟节点的指针。我们使用了`save`和`load`函数来将家族信息存储到文件中,并从文件中加载家族信息。`print_node`和`print_tree`函数用于打印家族信息和家族树。`find_node`函数用于查找某个家族成员节点。`find_sons`和`find_parents`函数用于查找某个家族成员的儿子和父母。
在主函数中,我们设置了一个菜单,可以进行添加家族成员、打印家族树、查找儿子和查找父母等操作。我们使用了`find_node`函数来查找某个家族成员节点,然后根据需要调用`find_sons`和`find_parents`函数来查找其儿子和父母。每当我们添加或修改家族成员信息时,都会将其保存到文件中。
相关推荐
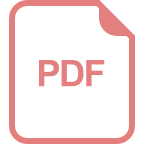
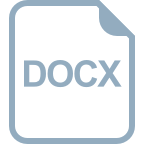
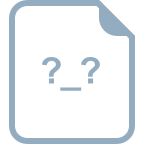










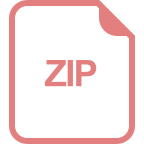
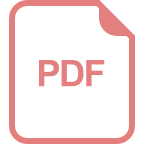
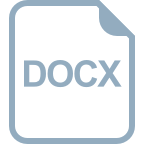
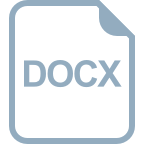
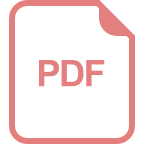