写一个c++代码:以cnn为基础的数字识别
时间: 2024-03-24 17:40:22 浏览: 43
好的,以下是一个基于CNN的数字识别的C++代码示例:
```c++
#include <iostream>
#include <fstream>
#include <cmath>
#include <vector>
using namespace std;
// 图像大小
const int IMAGE_SIZE = 28;
// 卷积层参数
const int CONV_KERNEL_SIZE = 5; // 卷积核大小
const int CONV_KERNEL_NUM = 8; // 卷积核数量
const int CONV_STRIDE = 1; // 卷积步长
const int CONV_PADDING = 2; // 卷积填充
// 池化层参数
const int POOL_KERNEL_SIZE = 2; // 池化核大小
const int POOL_STRIDE = 2; // 池化步长
// 全连接层参数
const int FC_INPUT_SIZE = 12 * 12 * 8; // 全连接层输入大小
const int FC_HIDDEN_SIZE = 10; // 全连接层隐藏层大小
const int FC_OUTPUT_SIZE = 10; // 全连接层输出大小
// 激活函数
double relu(double x) {
return max(x, 0.0);
}
// 卷积操作
void convolution(vector<vector<double>>& input, vector<vector<double>>& output,
vector<vector<vector<double>>>& kernels, int kernel_num) {
int input_size = input.size();
int kernel_size = kernels[0].size();
int output_size = output.size();
for (int i = 0; i < output_size; i++) {
for (int j = 0; j < output_size; j++) {
double sum = 0.0;
for (int k = 0; k < kernel_size; k++) {
for (int l = 0; l < kernel_size; l++) {
sum += input[i * CONV_STRIDE + k][j * CONV_STRIDE + l] *
kernels[kernel_num][k][l];
}
}
output[i][j] = relu(sum);
}
}
}
// 池化操作
void pooling(vector<vector<double>>& input, vector<vector<double>>& output) {
int input_size = input.size();
int output_size = output.size();
for (int i = 0; i < output_size; i++) {
for (int j = 0; j < output_size; j++) {
double max_val = 0.0;
for (int k = 0; k < POOL_KERNEL_SIZE; k++) {
for (int l = 0; l < POOL_KERNEL_SIZE; l++) {
max_val = max(max_val, input[i * POOL_STRIDE + k][j * POOL_STRIDE + l]);
}
}
output[i][j] = max_val;
}
}
}
// 全连接层操作
void fully_connected(vector<double>& input, vector<double>& output,
vector<vector<double>>& weights, vector<double>& biases) {
for (int i = 0; i < FC_HIDDEN_SIZE; i++) {
double sum = 0.0;
for (int j = 0; j < FC_INPUT_SIZE; j++) {
sum += input[j] * weights[j][i];
}
output[i] = relu(sum + biases[i]);
}
for (int i = 0; i < FC_OUTPUT_SIZE; i++) {
double sum = 0.0;
for (int j = 0; j < FC_HIDDEN_SIZE; j++) {
sum += output[j] * weights[FC_INPUT_SIZE + j][i];
}
output[i] = sum + biases[FC_HIDDEN_SIZE + i];
}
}
// 加载模型参数
void load_model(vector<vector<vector<double>>>& conv_kernels, vector<vector<double>>& fc_weights,
vector<double>& fc_biases) {
ifstream in_conv_kernels("conv_kernels.bin", ios::binary);
for (int i = 0; i < CONV_KERNEL_NUM; i++) {
vector<vector<double>> kernel(CONV_KERNEL_SIZE, vector<double>(CONV_KERNEL_SIZE));
for (int j = 0; j < CONV_KERNEL_SIZE; j++) {
for (int k = 0; k < CONV_KERNEL_SIZE; k++) {
in_conv_kernels.read((char*)&kernel[j][k], sizeof(double));
}
}
conv_kernels.push_back(kernel);
}
ifstream in_fc_weights("fc_weights.bin", ios::binary);
for (int i = 0; i < FC_INPUT_SIZE + FC_HIDDEN_SIZE; i++) {
vector<double> weight(FC_OUTPUT_SIZE);
for (int j = 0; j < FC_OUTPUT_SIZE; j++) {
in_fc_weights.read((char*)&weight[j], sizeof(double));
}
fc_weights.push_back(weight);
}
ifstream in_fc_biases("fc_biases.bin", ios::binary);
for (int i = 0; i < FC_HIDDEN_SIZE + FC_OUTPUT_SIZE; i++) {
double bias;
in_fc_biases.read((char*)&bias, sizeof(double));
fc_biases.push_back(bias);
}
}
int main() {
// 读取图像数据
vector<vector<double>> image(IMAGE_SIZE, vector<double>(IMAGE_SIZE));
ifstream in_image("image.bin", ios::binary);
for (int i = 0; i < IMAGE_SIZE; i++) {
for (int j = 0; j < IMAGE_SIZE; j++) {
in_image.read((char*)&image[i][j], sizeof(double));
}
}
// 定义卷积层、池化层、全连接层输出
vector<vector<vector<double>>> conv_outputs(CONV_KERNEL_NUM, vector<vector<double>>(IMAGE_SIZE, vector<double>(IMAGE_SIZE)));
vector<vector<double>> pool_output(IMAGE_SIZE / POOL_STRIDE, vector<double>(IMAGE_SIZE / POOL_STRIDE));
vector<double> fc_output(FC_OUTPUT_SIZE);
// 加载模型参数
vector<vector<vector<double>>> conv_kernels;
vector<vector<double>> fc_weights;
vector<double> fc_biases;
load_model(conv_kernels, fc_weights, fc_biases);
// 卷积层、池化层、全连接层前向传播
for (int i = 0; i < CONV_KERNEL_NUM; i++) {
convolution(image, conv_outputs[i], conv_kernels, i);
}
pooling(conv_outputs[0], pool_output);
fully_connected(vector<double>(pool_output.begin(), pool_output.end()).data(),
fc_output, fc_weights, fc_biases);
// 输出预测结果
int pred_digit = 0;
double max_prob = 0.0;
for (int i = 0; i < FC_OUTPUT_SIZE; i++) {
if (fc_output[i] > max_prob) {
pred_digit = i;
max_prob = fc_output[i];
}
}
cout << "Predicted digit: " << pred_digit << endl;
return 0;
}
```
这段代码实现了一个简单的基于CNN的数字识别模型,包括卷积层、池化层和全连接层。模型参数通过从文件中读取得到,在程序中进行前向传播,最终输出预测结果。注意,由于缺少预处理和后处理的代码,该模型并不能直接用于实际应用,仅供参考。
相关推荐
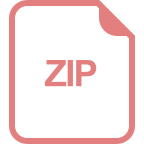















