dask.compute()函数怎么使用distributed并行计算?
时间: 2024-05-13 14:15:04 浏览: 199
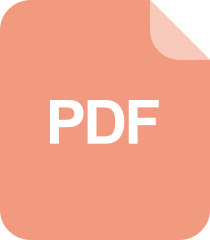
dask Documentation
要使用Dask进行分布式计算,需要先启动一个Dask集群。可以使用以下代码启动一个本地集群:
```python
from dask.distributed import Client, LocalCluster
cluster = LocalCluster()
client = Client(cluster)
```
然后,可以将需要计算的任务定义为Dask图,并使用`dask.compute()`函数进行计算。对于使用分布式计算的任务,需要在`dask.compute()`中传递一个`Client`对象,以便Dask将计算任务分配到集群中的不同工作节点上:
```python
result = dask.compute(task1, task2, task3, client=client)
```
其中,`task1`,`task2`和`task3`是需要计算的任务。`dask.compute()`会自动将这些任务转换为一个Dask图,并将其分配到集群中的不同工作节点上进行计算。`result`将包含每个任务的计算结果。
需要注意的是,使用分布式计算可能会带来一些额外的开销,如数据传输和通信开销。因此,建议仅在计算任务较大而单个计算节点无法满足要求时使用分布式计算。
阅读全文
相关推荐
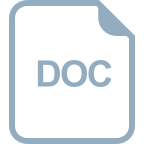
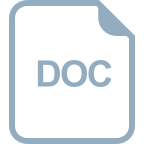


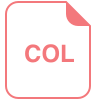
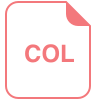
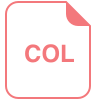
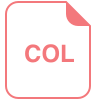
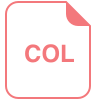
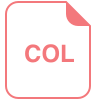
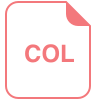
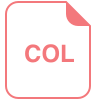
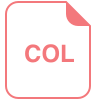
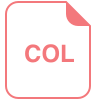
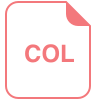
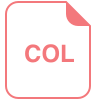
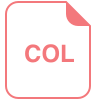