opencvsharp找到点云集合的线段交点
时间: 2023-08-14 22:16:17 浏览: 103
要找到点云集合的线段交点,可以使用OpenCVSharp库中的Subdiv2D类来进行Delaunay三角剖分,然后使用findIntersection函数找到线段交点。以下是一个示例代码:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using OpenCvSharp;
static void Main(string[] args)
{
// 生成随机点云
List<Point2f> points = new List<Point2f>();
Random rand = new Random();
for (int i = 0; i < 50; i++)
{
float x = rand.Next(0, 400);
float y = rand.Next(0, 400);
points.Add(new Point2f(x, y));
}
// Delaunay三角剖分
Subdiv2D subdiv = new Subdiv2D(new Rect(0, 0, 400, 400));
foreach (Point2f p in points)
{
subdiv.Insert(p);
}
// 找到所有线段
List<VoronoiEdge> edges = new List<VoronoiEdge>();
subdiv.GetEdgeList(edges);
// 找到所有线段的交点
List<Point2f> intersectionPoints = new List<Point2f>();
for (int i = 0; i < edges.Count; i++)
{
for (int j = i + 1; j < edges.Count; j++)
{
Point2f? intersectionPoint = findIntersection(edges[i], edges[j]);
if (intersectionPoint != null)
{
intersectionPoints.Add(intersectionPoint.Value);
}
}
}
// 输出所有交点的坐标
foreach (Point2f p in intersectionPoints)
{
Console.WriteLine($"Intersection Point: ({p.X}, {p.Y})");
}
}
// 找到两条线段的交点
static Point2f? findIntersection(VoronoiEdge e1, VoronoiEdge e2)
{
float x1 = e1.Vx1;
float y1 = e1.Vy1;
float x2 = e1.Vx2;
float y2 = e1.Vy2;
float x3 = e2.Vx1;
float y3 = e2.Vy1;
float x4 = e2.Vx2;
float y4 = e2.Vy2;
float denominator = (y4 - y3) * (x2 - x1) - (x4 - x3) * (y2 - y1);
if (denominator == 0)
{
return null;
}
float ua = ((x4 - x3) * (y1 - y3) - (y4 - y3) * (x1 - x3)) / denominator;
float ub = ((x2 - x1) * (y1 - y3) - (y2 - y1) * (x1 - x3)) / denominator;
if (ua < 0 || ua > 1 || ub < 0 || ub > 1)
{
return null;
}
float intersectionX = x1 + ua * (x2 - x1);
float intersectionY = y1 + ua * (y2 - y1);
return new Point2f(intersectionX, intersectionY);
}
```
此代码将生成50个随机点,并使用Subdiv2D类进行Delaunay三角剖分。然后,它将找到所有线段,并使用findIntersection函数找到线段交点。最后,它将输出所有交点的坐标。你可以根据需要进行修改以适应你的应用程序。
阅读全文
相关推荐










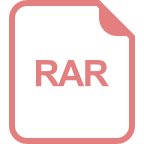
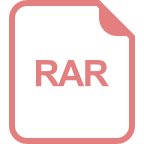



