C# WEB API Autofac 批量依赖自动注入
时间: 2023-12-06 15:45:15 浏览: 131
在使用 Autofac 进行批量依赖注入时,可以使用 Autofac 的 `RegisterAssemblyTypes` 方法实现。
首先,需要在程序集中标记需要注入的类型,可以使用 `Autofac.Module` 类进行实现。例如:
```csharp
using Autofac;
public class MyModule : Autofac.Module
{
protected override void Load(ContainerBuilder builder)
{
builder.RegisterType<MyService>().As<IMyService>();
builder.RegisterType<MyRepository>().As<IMyRepository>();
// 注册其他需要注入的类型
}
}
```
然后,在 `Global.asax.cs` 文件中,使用 `ContainerBuilder` 类创建容器,并把需要注入的模块注册进去。例如:
```csharp
using Autofac;
using Autofac.Integration.WebApi;
using System.Reflection;
using System.Web.Http;
public class WebApiApplication : System.Web.HttpApplication
{
protected void Application_Start()
{
var builder = new ContainerBuilder();
builder.RegisterApiControllers(Assembly.GetExecutingAssembly());
builder.RegisterAssemblyModules(Assembly.GetExecutingAssembly());
var container = builder.Build();
GlobalConfiguration.Configuration.DependencyResolver = new AutofacWebApiDependencyResolver(container);
}
}
```
这样,就可以实现批量依赖注入了。在需要使用注入的类型时,可以通过构造函数注入或属性注入的方式获取实例。例如:
```csharp
public class MyController : ApiController
{
private readonly IMyService _myService;
public MyController(IMyService myService)
{
_myService = myService;
}
[HttpGet]
public IHttpActionResult MyAction()
{
// 使用 MyService
return Ok();
}
}
```
阅读全文
相关推荐
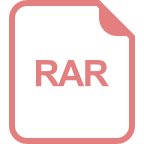
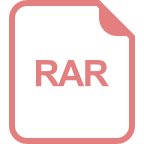
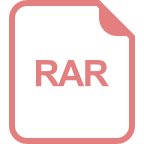
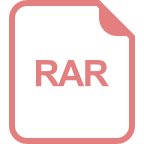
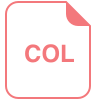

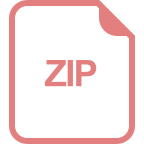
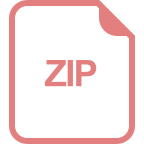
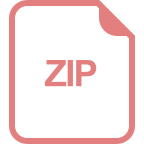
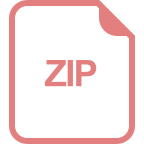
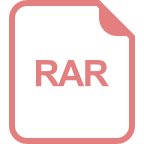
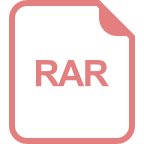
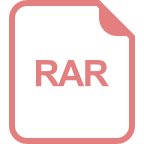
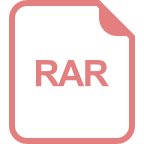
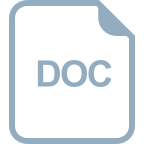
