python求语音信号的频谱包络
时间: 2023-09-05 19:12:55 浏览: 206
可以使用python中的scipy库来求取语音信号的频谱包络。具体步骤如下:
1. 读取语音信号,可以使用Python中的wave库或者pydub库。
2. 对语音信号进行预处理,比如进行预加重、分帧、加窗等操作,可以使用Python中的librosa库。
3. 对每一帧信号进行傅里叶变换,得到频谱,可以使用Python中的numpy库或者scipy库中的fft函数。
4. 对每一帧频谱进行平滑处理,得到频谱包络,可以使用Python中的savgol_filter函数或者medfilt函数。
5. 可以将每一帧的频谱包络拼接起来,得到整个语音信号的频谱包络。
下面是一个示例代码:
```python
import numpy as np
from scipy import signal
import matplotlib.pyplot as plt
import librosa
# 读取语音信号
y, sr = librosa.load('speech.wav', sr=None)
# 预处理
pre_emphasis = 0.97
y_pre = signal.lfilter([1, -pre_emphasis], [1], y)
frame_size = int(sr * 0.025)
frame_stride = int(sr * 0.01)
frames = librosa.util.frame(y_pre, frame_length=frame_size, hop_length=frame_stride)
frames *= np.hamming(frame_size)[:, np.newaxis]
# 计算每一帧的频谱包络
envelopes = []
for i in range(frames.shape[1]):
frame = frames[:, i]
# 傅里叶变换
spectrum = np.abs(np.fft.fft(frame))[:frame_size // 2]
# 平滑处理
envelope = signal.savgol_filter(spectrum, 11, 3)
envelopes.append(envelope)
# 拼接得到整个语音信号的频谱包络
envelope = np.concatenate(envelopes)
# 绘制频谱包络
plt.plot(envelope)
plt.xlabel('Frequency')
plt.ylabel('Envelope')
plt.show()
```
其中,示例代码使用了预加重和汉明窗加窗,使用了savgol_filter函数进行平滑处理。你也可以根据自己的需求进行调整。
阅读全文
相关推荐

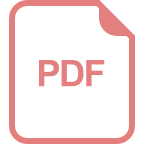
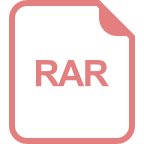
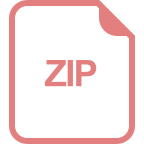
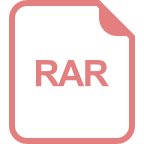
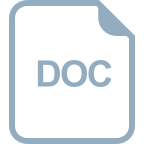
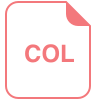
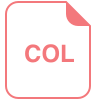
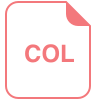
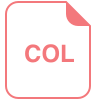
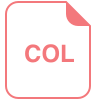
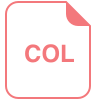
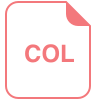
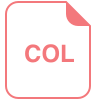
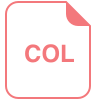
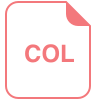