java代码配置文件修改MySQL、Redis连接信息怎么修改,我要修改地址、端口、数据库名称、数据库用户名和密码等,MySQL、Redis都要修改为这种格式
时间: 2023-11-24 11:06:33 浏览: 30
对于MySQL连接信息的修改,可以在Java中使用JDBC连接数据库。修改MySQL连接信息主要需要修改以下几个参数:
1. URL:连接MySQL数据库的URL,格式为:jdbc:mysql://host:port/database,其中host为MySQL服务器主机名,port为MySQL服务器端口号,database为要连接的数据库名称。
2. 用户名和密码:连接MySQL数据库需要提供正确的用户名和密码。
以下是示例代码:
```java
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class MySQLConnection {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydatabase";
String username = "myusername";
String password = "mypassword";
Connection conn = null;
try {
conn = DriverManager.getConnection(url, username, password);
System.out.println("Connected to MySQL database!");
} catch (SQLException e) {
System.out.println("Failed to connect to MySQL database");
e.printStackTrace();
} finally {
if (conn != null) {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
}
```
对于Redis连接信息的修改,可以使用Jedis库连接Redis数据库。修改Redis连接信息主要需要修改以下几个参数:
1. Host和Port:Redis服务器的主机名和端口号。
2. Password:连接Redis数据库需要提供正确的密码。
以下是示例代码:
```java
import redis.clients.jedis.Jedis;
public class RedisConnection {
public static void main(String[] args) {
String host = "localhost";
int port = 6379;
String password = "mypassword";
Jedis jedis = null;
try {
jedis = new Jedis(host, port);
jedis.auth(password);
System.out.println("Connected to Redis database!");
} catch (Exception e) {
System.out.println("Failed to connect to Redis database");
e.printStackTrace();
} finally {
if (jedis != null) {
jedis.close();
}
}
}
}
```
在实际使用中,你需要将以上示例代码中的连接信息修改为你自己的MySQL或Redis连接信息。
相关推荐
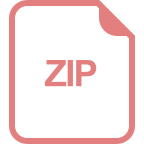
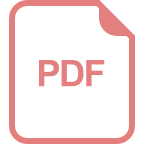
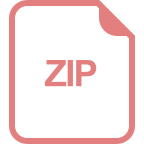














