import pandas as pd import pymysql # 连接到数据库 conn = pymysql.connect(host='localhost', user='user', password='password', database='database') # 获取所有表格的名称 cursor = conn.cursor() cursor.execute("SHOW TABLES") tables = cursor.fetchall() # 遍历所有表格 for table in tables: table_name = table[0] table_name_quoted = '' + table_name + '' # 检查是否存在名为'a'的列,如果不存在则添加'a'和'b'列 cursor.execute("SHOW COLUMNS FROM " + table_name_quoted + " LIKE 'a'") a_column = cursor.fetchone() if a_column is None: cursor.execute("ALTER TABLE " + table_name_quoted + " ADD COLUMN a DECIMAL(10,2)") cursor.execute("ALTER TABLE " + table_name_quoted + " ADD COLUMN b DECIMAL(10,2)") conn.commit() # 查询net_mf_amount列的数据 query = "SELECT trade_date, net_mf_amount FROM " + table_name_quoted + " ORDER BY trade_date DESC" df = pd.read_sql_query(query, conn) # 计算a和b列 a_column = [] b_column = [] for i in range(len(df)): if i == 0: a_column.append(None) b_column.append(None) else: if pd.notnull(df.iloc[i]['net_mf_amount']) and pd.notnull(df.iloc[i-1]['net_mf_amount']): if i-2 >= 0: if pd.notnull(df.iloc[i-2]['net_mf_amount']): a = df.iloc[i]['net_mf_amount'] - df.iloc[i-1]['net_mf_amount'] b = df.iloc[i]['net_mf_amount'] - df.iloc[i-2]['net_mf_amount'] a_column.append(a) b_column.append(b) else: j = i-3 while j >= 0: if pd.notnull(df.iloc[j]['net_mf_amount']): a = df.iloc[i]['net_mf_amount'] - df.iloc[i-1]['net_mf_amount'] b = df.iloc[i]['net_mf_amount'] - df.iloc[j]['net_mf_amount'] a_column.append(a) b_column.append(b) break j -= 1 else: a = df.iloc[i]['net_mf_amount'] - df.iloc[i-1]['net_mf_amount'] b = None a_column.append(a) b_column.append(b) else: a_column.append(None) b_column.append(None) # 将结果保存到数据库 for i in range(len(df)): cursor.execute("UPDATE " + table_name_quoted + " SET a=%s, b=%s WHERE trade_date=%s", (a_column[i], b_column[i], df.iloc[i]['trade_date'])) conn.commit() # 关闭连接 cursor.close() conn.close() 报错/Users/ljy/stock/daily_analyse.py:65: UserWarning: pandas only supports SQLAlchemy connectable (engine/connection) or database string URI or sqlite3 DBAPI2 connection. Other DBAPI2 objects are not tested. Please consider using SQLAlchemy. df = pd.read_sql_query(query, conn)
时间: 2024-02-11 13:08:11 浏览: 27
这段代码的报错和之前的一样,是因为使用的连接对象 conn 不是 SQLAlchemy 连接对象或者 SQLite3 的 DBAPI2 连接对象。你可以将该连接对象转换为 SQLAlchemy 连接对象或者使用数据库字符串 URI 来连接数据库。将连接数据库的代码修改如下:
```
import pandas as pd
from sqlalchemy import create_engine
# 连接到数据库
engine = create_engine('mysql+pymysql://user:password@localhost/database')
# 获取所有表格的名称
with engine.connect() as conn, conn.begin():
tables = conn.execute("SHOW TABLES").fetchall()
# 遍历所有表格
for table in tables:
table_name = table[0]
table_name_quoted = '`' + table_name + '`'
# 检查是否存在名为'a'的列,如果不存在则添加'a'和'b'列
with engine.connect() as conn, conn.begin():
a_column = conn.execute("SHOW COLUMNS FROM " + table_name_quoted + " LIKE 'a'").fetchone()
if a_column is None:
conn.execute("ALTER TABLE " + table_name_quoted + " ADD COLUMN a DECIMAL(10,2)")
conn.execute("ALTER TABLE " + table_name_quoted + " ADD COLUMN b DECIMAL(10,2)")
# 查询net_mf_amount列的数据
query = "SELECT trade_date, net_mf_amount FROM " + table_name_quoted + " ORDER BY trade_date DESC"
df = pd.read_sql_query(query, engine)
# 计算a和b列
a_column = []
b_column = []
for i in range(len(df)):
if i == 0:
a_column.append(None)
b_column.append(None)
else:
if pd.notnull(df.iloc[i]['net_mf_amount']) and pd.notnull(df.iloc[i-1]['net_mf_amount']):
if i-2 >= 0:
if pd.notnull(df.iloc[i-2]['net_mf_amount']):
a = df.iloc[i]['net_mf_amount'] - df.iloc[i-1]['net_mf_amount']
b = df.iloc[i]['net_mf_amount'] - df.iloc[i-2]['net_mf_amount']
a_column.append(a)
b_column.append(b)
else:
j = i-3
while j >= 0:
if pd.notnull(df.iloc[j]['net_mf_amount']):
a = df.iloc[i]['net_mf_amount'] - df.iloc[i-1]['net_mf_amount']
b = df.iloc[i]['net_mf_amount'] - df.iloc[j]['net_mf_amount']
a_column.append(a)
b_column.append(b)
break
j -= 1
else:
a = df.iloc[i]['net_mf_amount'] - df.iloc[i-1]['net_mf_amount']
b = None
a_column.append(a)
b_column.append(b)
else:
a_column.append(None)
b_column.append(None)
# 将结果保存到数据库
with engine.connect() as conn, conn.begin():
for i in range(len(df)):
conn.execute("UPDATE " + table_name_quoted + " SET a=%s, b=%s WHERE trade_date=%s", (a_column[i], b_column[i], df.iloc[i]['trade_date']))
# 关闭连接
engine.dispose()
```
注意:上述代码中的 user, password 和 database 分别对应的是你自己的用户名、密码和数据库名,需要进行修改。
相关推荐
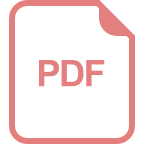
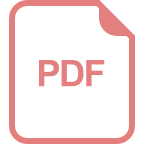















